In previous lessons, we learned how to turn an LED on and off by Arduino programming. It is also possible to control the brightness of your LED as well. There are 6 digital pins marked with “~” on your micro-controller. This means that these pins can use a PWM signal. We will build an LED breathing light effect by using PWM control to gradually make the LED brighter and dimmer.
COMPONENT LIST
HARDWARE
fig 1 Fading Light Circuit
*The wiring diagram resembles that of Project [Blinking A LED], but please pay attention to the specific pins being connected. Refer to the circuit diagram above for accurate wiring instructions.
CODE
Sample code:
// Project - Fading light
int ledPin = 10;
void setup() {
pinMode(ledPin, OUTPUT);
// Set the LED pin as an OUTPUT
}
void loop(){
fadeOn(1000, 5);
// Fade the LED in over 1000 milliseconds, incrementing by 5
fadeOff(1000, 5);
// Fade the LED out over 1000 milliseconds, decrementing by 5
}
void fadeOn(unsigned int time, int increment) {
for (byte value = 0; value < 255; value += increment) {
// Loop from 0 to 255, incrementing by 'increment'
analogWrite(ledPin, value);
// Set the brightness of the LED to the current value
delay(time / (255 / increment));
// Delay for a fraction of the total time based on the increment
}
}
void fadeOff(unsigned int time, int decrement) {
for (byte value = 255; value > 0; value -= decrement) {
// Loop from 255 to 0, decrementing by 'decrement'
analogWrite(ledPin, value);
// Set the brightness of the LED to the current value
delay(time / (255 / decrement));
// Delay for a fraction of the total time based on the decrement
}
}
You will see the LED getting brighter and darker constantly after uploading the code.
CODE REVIEW
Most of the knowledge in the sample code has already been covered in our previous studies, such as initializing variable declarations, setting pins, setting up the for loop, and the function call.
In the main code, we only use 2 functions. You will have a clear idea after checking one of them as below.
void fadeOn(unsigned int time, int increment) {
for (byte value = 0; value < 255; value += increment) {
analogWrite(ledPin, value);
delay(time / (255 / increment));
}
}
The fadeOn() function has 2 parameters, int time for time and int increment for the increasing values. There is a for() statement that repeats the program. The condition is “value < 255” and the amount of brightness increase is decided by increment.
This is a new command in the for() function:
analogWrite(ledPin, value);
How can we send analog values to a digital pin? We use pins marked with a "~" on the end, such as D3, D5, D6, D9, D10 and D11, to output a variable amount of power to the LED. This technique of controlling power is known as "Pulse Width Modulation" or PWM for short.
The format of the analogWrite command is as below:
The analogWrite() function is to assign the PWM pin an analog value between 0 and 255.
PWM
Roughly every 1/500 of a second, the PWM pins output a pulse using digital signals. By controlling the length of on and off, it creates an equivalent effect of carrying out voltage between 0 volts and 5 volts. The length of pulse is called “pulse width” so PWM refers to pulse width modulation.
Let's take a closer look at PWM.

The green line is the cycle of the pulse. The percentage of length of high voltage and low voltage according to the value of analogWrite() function is known as the “Duty Cycle”. The duty cycle of the first pulse is 0. So the value is 0 and the brightness of the LED is 0, equivalent to off. The longer the signal is HIGH, the brighter the LED is. The duty cycle of the last pulse is 100%. So the value is 255 and the brightness of the LED is 255. Likewise, 50% is half brightness and 25% is relatively dimmer.
PWM is widely used in controlling light brightness. We also use it to control the rotating speed of motors, such as the wheels of vehicles powered by motors.
This chapter is over! Although we have used the same hardware as in Project[Blinking A LED], Arduino is running a different program, resulting in a completely different effect.
We think that the Arduino is amazing and we hope you love it too!
EXERCISE
1. Create a flickering flame effect using LEDs by controlling the value of PWM at random. Cover it with paper and it will become a little lamp at night.
Materials:
1 x red LED
2 x yellow LED
1 x 220Ω resistor
Use the “random” command to adjust the brightness level. We suggest you initialize a brightness level first, and let it change within a random value, such as random(-60, 60)+135. This way, the LED can change within a small amount just like a real flame!
You can check the references below for more explanations of various commands.
2. Try a more challenging project: Control the LED with 2 buttons, one to make it brighter, and the other to make it dimmer.
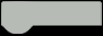