Imagine being able to effortlessly control the lights with just a wave of your hand. This not only adds convenience to daily life but also enhances the interactivity and enjoyment of smart home technology. There are various gesture recognition technologies available. In this project, we will use two light sensors to achieve gesture control by detecting changes in light blockage at different hand positions.
COMPONENT LIST

HARDWARE

fig 1 Gesture-Controlled Light
CODE
Sample code :
int ledPin = 13; // Define the LED pin 13
int lightSensor1 = 0; // Define the left light sensor pin A0
int lightSensor2 = 1; // Define the right light sensor pin A1
int cali1 = 0; // Calibration variable for left light sensor
int cali2 = 0; // Calibration variable for right light sensor
bool isOn = false; // LED state control variable
void setup() {
Serial.begin(9600); // Initialize serial communication
pinMode(ledPin, OUTPUT); // Set the LED pin as output
/*Calibrate light sensors: read data 10 times and average as ambient light baseline*/
for (int i = 0; i < 10; i++) {
cali1 += analogRead(lightSensor1);
cali2 += analogRead(lightSensor2);
delay(500);
}
cali1 = (cali1 / 10);
cali2 = (cali2 / 10);
Serial.print("Ambient light: ");
Serial.print(cali1);
Serial.print(" , ");
Serial.println(cali2);
// Blink the LED twice to indicate the start of the program
for (int i = 0; i < 2; i++) {
digitalWrite(ledPin, HIGH);
delay(1000);
digitalWrite(ledPin, LOW);
delay(1000);
}
Serial.println("Start");
}
void loop() {
int sensorVal1 = analogRead(lightSensor1);
// Read the value of the left light sensor
int sensorVal2 = analogRead(lightSensor2);
// Read the value of the right light sensor
int threshold = 30;
// Set the threshold value for gesture detection
Serial.print("L: ");
Serial.print(sensorVal1);
Serial.print(" R: ");
Serial.println(sensorVal2);
// Determine gesture direction and control the LED
if ((sensorVal1 > (cali1 + threshold)) && !(sensorVal2 > (cali2 + threshold))) {
isOn = true;
}
if (!(sensorVal1 > (cali1 + threshold)) && (sensorVal2 > (cali2 + threshold))) {
isOn = false;
}
if (isOn) {
digitalWrite(ledPin, HIGH); // Turn on the LED
Serial.println("Turn On");
} else {
digitalWrite(ledPin, LOW); // Turn off the LED
Serial.println("Turn Off");
}
delay(100); // Delay 100 milliseconds to avoid frequent readings
}
Please place the device in a stable light environment without any shadows. After uploading the program, it will automatically calibrate for 5 seconds to detect the current light values. Once calibration is complete, the LED will blink twice to indicate the start of the program. Waving your hand to the left will turn on the light, and waving your hand to the right will turn off the light. If the performance is not satisfactory, you can refer to the readings printed on the serial monitor and adjust the threshold settings accordingly.
CODE REVIEW
At the beginning of the program, define the pins for the LED and the two photoresistors.
Then, create two variables named cali to record the initial readings of the photoresistors.

Next, we introduce a new variable type, bool, which represents a Boolean variable. It has only two possible values: true and false, typically used to represent the truth of conditions or the state of a switch. Here, we define a Boolean variable named isOn to record the current on/off state of the LED.

The readings of the photoresistors vary depending on their position and the time of day. Therefore, in the setup phase of the program, we need to perform automatic calibration to obtain the baseline readings of the sensors.
for (int i = 0; i < 10; i++) {
cali1 += analogRead(lightSensor1);
cali2 += analogRead(lightSensor2);
delay(500);
}
In the For Loop, the analog values of the photoresistors were read 10 times with a 500- millisecond interval, and the results were accumulated
into the variables cali1 and cali2.
After the loop concludes, calculate the average values of the left and right photoresistors. These averages will serve as calibrated baseline values for subsequent light comparisons and decisions.
Once calibrated, print the average values on the serial monitor and blink the LED twice to indicate the program's start.
In the loop function, we'll compare the light values and control the LED.
First, set up two variables to record the real-time readings of the photoresistors.

From previous lessons on photoresistors, we've learned that higher readings correspond to darker light conditions. When gestures occur, shadows cast on different parts of the photoresistors cause their readings to rise. Therefore, we can distinguish left and right waving gestures by monitoring changes in the readings of the photoresistors on
both sides. Adjusting the threshold variable allows us to refine the sensitivity of this detection process.

When the reading of the left photoresistor surpasses its baseline value plus the threshold, it indicates obstruction on the left side.

However, relying solely on the left-side reading is insufficient; it needs to be combined with the rightside photoresistor reading for accurate assessment.
When the left side is obstructed while the right side is not, it indicates a gesture waving from right to left.

In this line of code, ! serves as the logical NOT operator, which reverses the value of the internal expression. && acts as the logical AND operator, checking if both expressions are true simultaneously. If the condition is satisfied, isOn is set to true, turning on the LED. Otherwise, isOn is set to false.

Finally, we use the current value of isOn to decide whether to turn the LED on or off. Here, if (isOn) is a shorthand for if (isOn == true).
if (isOn) {
digitalWrite(ledPin, HIGH);
Serial.println("Turn On");
} else {
digitalWrite(ledPin, LOW);
Serial.println("Turn Off");
}
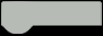