An 8-segment LED is a form of electronic display device for displaying decimal numerals. It is an alternative to more complex dot matrix displays. By controlling each LED in each segment connected to a digital pin, numbers can be displayed on this LED.
COMPONENT LIST

HARDWARE
The 8-segment LED has 10 pins. The 5 pins on the upper position are connected to digital pin 2 to digital pin 5. The other 5 pins on the lower position with decimal point are connected to digital pins 6 to 9. 8-resistors are included to limit the current for the LEDs.

fig 1 Infrared Controlled LED Matrix
CODE_1
Sample code1:
// Project - Infrared Controlled LED Matrix_1
void setup(){
for(int pin = 2 ; pin <= 9 ; pin++){
// Set digital pins 2 to 9 to OUTPUT mode
pinMode(pin, OUTPUT);
digitalWrite(pin, HIGH);
// Initialize all pins to HIGH (assuming common anode display)
}
}
void loop() {
// Display digit 0
int n0[8]={0,0,0,1,0,0,0,1};
for(int pin = 2; pin <= 9 ; pin++){
/* Digital pins 2 to 9 display values from array n0[8] sequentially */
digitalWrite(pin,n0[pin-2]);
}
delay(500);
// Display digit 1
int n1[8]={0,1,1,1,1,1,0,1};
for(int pin = 2; pin <= 9 ; pin++){
/* Digital pins 2 to 9 display values from array n1[8] sequentially */
digitalWrite(pin,n1[pin-2]);
}
delay(500);
// Display digit 2
int n2[8]={0,0,1,0,0,0,1,1};
for(int pin = 2; pin <= 9 ; pin++){
/* Digital pins 2 to 9 display values from array n2[8] sequentially */
digitalWrite(pin,n2[pin-2]);
}
delay(500);
// Display digit 3
int n3[8]={0,0,1,0,1,0,0,1};
for(int pin = 2; pin <= 9 ; pin++){ // Digital pins 2 to 9 display values from array n3[8] sequentially
digitalWrite(pin,n3[pin-2]);
}
delay(500);
// Display digit 4
int n4[8]={0,1,0,0,1,1,0,1};
for(int pin = 2; pin <= 9 ; pin++){
/* Digital pins 2 to 9 display values from array n4[8] sequentially */
digitalWrite(pin,n4[pin-2]);
}
delay(500);
// Display digit 5
int n5[8]={1,0,0,0,1,0,0,1};
for(int pin = 2; pin <= 9 ; pin++){
/* Digital pins 2 to 9 display values from array n5[8] sequentially */
digitalWrite(pin,n5[pin-2]);
}
delay(500);
// Display digit 6
int n6[8]={1,0,0,0,0,0,0,1};
for(int pin = 2; pin <= 9 ; pin++){
/* Digital pins 2 to 9 display values from array n6[8] sequentially */
digitalWrite(pin,n6[pin-2]);
}
delay(500);
// Display digit 7
int n7[8]={0,0,1,1,1,1,0,1};
for(int pin = 2; pin <= 9 ; pin++){
/* Digital pins 2 to 9 display values from array n7[8] sequentially */
digitalWrite(pin,n7[pin-2]);
}
delay(500);
// Display digit 8
int n8[8]={0,0,0,0,0,0,0,1};
for(int pin = 2; pin <= 9 ; pin++){ /* Digital pins 2 to 9 display values from array n8[8] sequentially */
digitalWrite(pin,n8[pin-2]);
}
delay(500);
// Display digit 9
int n9[8]={0,0,0,0,1,0,0,1};
for(int pin = 2; pin <= 9 ; pin++){ /* Digital pins 2 to 9 display values from array n9[8] sequentially */
digitalWrite(pin,n9[pin-2]);
}
delay(500);
}
HARDWARE REVIEW
The 8-segment display has 10 pins: a, b, c, d, e, f, g and DP segments(decimal point). Each is connected to digital pins. By controlling each LED on the segment connected, numbers can be displayed on this LED. The other pin is a common pin that is connected to GND(common cathode) or 5V (common anode).

fig 2 Pin-out Instructions
Common Cathode and Common Anode Differences
All of the cathodes (negative terminals) or all of the anodes (positive terminals) of the segment LEDs are connected and brought out to a common pin; this is referred to as a "common cathode" or "common anode" device. The common pin of cathode LEDs is connected to GND. When you configure this pin HIGH, it triggers the LED to be ON. The common pin of anode LEDs is connected to 5V. When you configure this pin LOW, the LED is triggered to go OFF.
CODE REVIEW_2
The 8-segment LED is connected to 8 digital pins, so we need to define 8 digital pins with for loops to simplify things. Segment,b,a,f,g,e,d,c,DP correspond to digital pins from 2 to 9.
for(int pin = 2 ; pin <= 9 ; pin++){
// Set digital pins 2 to 9 to OUTPUT mode
pinMode(pin, OUTPUT);
digitalWrite(pin, HIGH);
// Initialize all pins to HIGH (assuming common anode display)
}
Set pins from 2 to 9 as OUTPUT and, initialize them HlGH. The main program is to display a number from 0 to 9. Let us take a look at the code of displaying 0.
int n1[8]={0,1,1,1,1,1,0,1};
Here we introduce the concept of an array. An array is a collection of variables that are accessed, with an index number. We declare an array of, int type, name it n0 and assign 8 values to, initialize it. Note that arrays are zero indexes. When declaring an array of type, one more element than, your initialization is required to hold the, required null character. Referring to the array, initialization above, the fourth element of the array, is at index 3(n0[3])and its value, is 1. The eighth element of the array is at index, 7(n0[7])and its value is 1. The 8 inside the square brackets (n0[8])means 8 elements.
After defining the array, we enter a for loop.
for(int pin = 2; pin <= 9 ; pin++){ // Digital pins 2 to 9 display values from array n1[8] sequentially
digitalWrite(pin,n1[pin-2]);
}
The for loop is to assign values HIGH/1 or LOW/0 to 2-9 pins. For example, when pin=2, n0[pin-2] is n0[0] which points to the first element of the array with value 0/LOW. So the "b" segment is on. When the for loop goes to pin=3, the "a" segment is on. When the for loops go to pin=4, the "f" segment is on, etc.
The loop process is as below:
pin=2’n0[0]=0’digitalWrite(2,0)’"b" segment ON
pin=3’n0[1]=0’digitalWrite(3,0)’"a" segment ON
pin=4’n0[2]=0’’digitalWrite(4,0)’"f" segment ON
pin=5’n0[3]=1’digitalWrite(5,1)’"g" segment OFF
pin=6’n0[4]=0’digitalWrite(6,0)’"e" segment ON
pin=7’n0[5]=0’digitalWrite(7,0)’"d" segment ON
pin=8’n0[6]=0’digitalWrite(8,0)’"c" segment ON
pin=9’n0[7]=1’digitalWrite(9,1)’"DP" segment OFF
Now it displays 0. It displays 1 to 9 based on the array. Just try to draw it out and the light-on-and-off status is clear.
An easier method is to be introduced based on the understanding of Sample code1. This will be introduced on the following page.
CODE_2
In this section, we will introduce an even simpler way to manipulate the loops for numbers 0 to 9 in the 8-segment LED. In the sketch above, we created 10 arrays to display 0 to 9. This is a one-dimensional array. What about creating a two-dimensional array to make it simpler?
Sample code2:
// Project - Infrared Controlled LED Matrix_2
int number[10][8] =
{
{0,0,0,1,0,0,0,1}, // Display 0
{0,1,1,1,1,1,0,1}, // Display 1
{0,0,1,0,0,0,1,1}, // Display 2
{0,0,1,0,1,0,0,1}, // Display 3
{0,1,0,0,1,1,0,1}, // Display 4
{1,0,0,0,1,0,0,1}, // Display 5
{1,0,0,0,0,0,0,1}, // Display 6
{0,0,1,1,1,1,0,1}, // Display 7
{0,0,0,0,0,0,0,1}, // Display 8
{0,0,0,0,1,0,0,1} // Display 9
};
void numberShow(int i){ // This function is used to display numbers
for(int pin = 2; pin <= 9 ; pin++){
digitalWrite(pin, number[i][pin - 2]);
}
}
void setup(){
for(int pin = 2 ; pin <= 9 ; pin++){
// Set digital pins 2 to 9 as OUTPUT mode
pinMode(pin, OUTPUT);
digitalWrite(pin, HIGH);
// Initialize all pins HIGH to ensure LEDs are OFF
}
}
void loop() {
for(int j = 0; j <= 9 ; j++){
numberShow(j);
// Call numberShow() function to display 0 to 9
delay(500);
// Wait for 500 milliseconds before displaying the next number
}
}
CODE REVIEW_2
The one-dimensional array is composed of elements and the two-dimensional array is composed with one-dimensional arrays.

In the first sketch, we manipulated 10 arrays, each with 8 elements corresponding to values in the segment from b to DP. In this sketch, we will manipulate 10 one-dimensional arrays together to form a two-dimensional array.


This is the two-dimensional array. It starts from the character "null". So number[0][0] is the first element in the first array whose value is 0. Number[9][7] is the eighth element in the eleventh array whose value is 1.


In the main program, the for loop manipulates the variable j to loop from 0 to 9. Once j is assigned a value, the numberShow0 function runs accordingly. The numberShow0 function runs as follows:
the numberShow() functions runs as below:
When the program begins, with j=0, numberShow(j) is numberShow(0). As it skips to the numberShow0 function, the value of i is 0 and the initial value of the pin is 2. The value of digitalWrite(2, number[0][0]) is set, whose value is 0. digitalWrite(2, 0) means to configure pin 2 as LOW, so the LED of segment b is OFF (assuming HIGH means LED is ON, but based on context, it's clearer to state LED state directly). Afterwards, the loop goes to pin=3, pin=4, until pin=9, when the whole for loop is finished and the number 0 displays on the 8-segment LED.
Compare how we displayed 0 in the first sketch.

The principle of assigning value for pins 2 to 9 to control segments from b to DP is the same.
When the numberShow(0) loop is finished, it goes back to the for loop function.
1 ’ numberShow(1) ’ i =1’ number[1][pin-2] ’ display 1
j=2 ’ numberShow(2) ’ i=2 ’ number[2][pin-2] ’ display 2
j=3 ’ numberShow(3) ’ i=3 ’ number[3][pin-2] ’ display 3
…¦…¦
j=9 ’ numberShow(9) ’ i=9 ’ number[9][pin-2] ’ displays 9
The above is the analysis for the codes. Just think about the differences between the one-dimensional array and the two-dimensional array and how the codes operate.
The combined application of the LED module and IR receiver is to be achieved after handling their operating principles. The Arduino compatible controller processes and delivers the signal transferred from the Mini remote controller to the IR receiver to the LED module.
Numbers from 0 to 9 on the mini remote controller are to be displayed on corresponding positions on the LED module. In addition, decreasing and increasing functions are feasible.
CODE_3
Sample code:
// Project - Infrared Controlled LED Matrix_3
#include <IRremote.h> // Include the IRremote library
int RECV_PIN = 11; // Define RECV_PIN as pin 11
IRrecv irrecv(RECV_PIN);
// Set RECV_PIN (pin 11) as the IR receiver pin
decode_results results;
// Define results variable to store IR decoding results
int currentNumber = 0; // Variable to store the current number
long codes[12] = {
// Array to store the IR codes emitted by the remote
0xFD30CF, 0xFD08F7, // 0, 1
0xFD8877, 0xFD48B7, // 2, 3
0xFD28D7, 0xFDA857, // 4, 5
0xFD6897, 0xFD18E7, // 6, 7
0xFD9867, 0xFD58A7, // 8, 9
0xFD20DF, 0xFD609F // Forward, Backward
};
int number[10][8] = {
// Array to store the 7-segment display patterns for digits
{0,0,0,1,0,0,0,1}, // 0
{0,1,1,1,1,1,0,1}, // 1
{0,0,1,0,0,0,1,1}, // 2
{0,0,1,0,1,0,0,1}, // 3
{0,1,0,0,1,1,0,1}, // 4
{1,0,0,0,1,0,0,1}, // 5
{1,0,0,0,0,0,0,1}, // 6
{0,0,1,1,1,1,0,1}, // 7
{0,0,0,0,0,0,0,1}, // 8
{0,0,0,0,1,0,0,1} // 9
};
void numberShow(int i) {
// Function to display a digit on the 7-segment display
for(int pin = 2; pin <= 9; pin++){
digitalWrite(pin, number[i][pin - 2]);
}
}
void setup(){
Serial.begin(9600);
// Start serial communication at 9600 baud
irrecv.enableIRIn(); // Enable IR decoding
for(int pin = 2; pin <= 9; pin++){
// Set digital pins 2 to 9 as OUTPUT
pinMode(pin, OUTPUT);
digitalWrite(pin, HIGH); /* Initialize all pins to HIGH (optional, depending on your setup) */
}
}
void loop() {
/* Check if a decoded signal is received and store it in the results variable */
if (irrecv.decode(&results)) {
for(int i = 0; i <= 11; i++){
// Check if the received code matches the codes for 0 to 9
if(results.value == codes[i] && i <= 9){
numberShow(i);
// Display the corresponding number on the 7-segment display
currentNumber = i;
// Assign the current displayed value to currentNumber
Serial.println(i);
// Print the number to the serial monitor
break;
}
/* Check if the received code is for decrement and the current number is not 0 */
else if(results.value == codes[10] && currentNumber != 0){
currentNumber--;
// Decrement the current number
numberShow(currentNumber);
// Display the decremented number
Serial.println(currentNumber);
// Print the decremented number to the serial monitor
break;
}
/* Check if the received code is for increment and the current number is not 9 */
else if(results.value == codes[11] && currentNumber != 9){
currentNumber++;
// Increment the current number
numberShow(currentNumber);
// Display the incremented number
Serial.println(currentNumber);
// Print the incremented number to the serial monitor
break;
}
}
/* Print the received IR code in hexadecimal to the serial monitor */
Serial.println(results.value, HEX);
irrecv.resume(); // Resume receiving the next signal
}
}
Codes downloaded, have a try to press some press buttons as shown in Fig. 3 to check the status of the LED module.

fig 3 Press Button Instructions
CODE REVIEW_3
The code below starts from the general declarations for the IRReceiver. Just copy Project[Infrared Controlled Light].

Here, there is an additional variable named currentNumber. This variable is for current value storage. A number increasing and decreasing is for the corresponding reference. An array is used to store these IR codes. "0x-" represents a hexadecimal number. Replaced IR codes work to realize additional functions via additional buttons on the remote controller.
First Step:
There are three conditions to consider:
1. 8-Segment LED Module: It should display numbers from 0 to 9 when the corresponding press buttons are pressed.
2. Backward Operation: Every time the Backward button is pressed, the displayed number decreases by 1 each time until it reaches 0.
3. Forward Operation: Every time the Forward button is pressed, the displayed number increases by 1 each time, until it reaches 9.
Get back to the codes for three conditions mentioned below:
if(results.value == codes[i] && i <= 9)
if(results.value == codes[10] && currentNumber != 0)
if(results.value == codes[11] && currentNumber != 9)
The first if() evaluates the first condition, displaying 0 to 9. The evaluation is based on whether data is received from results, and the values are from the IRcodes array, codes[0] to codes[9].
The second if() evaluates the second condition whether instructions from Backward are received (code[10] = 0xFD20DF) and the current number displayed is not 0.
The third if() evaluates the third condition whether instructions from Forward are received (code[11] = 0xFDA857) and the current number displayed is not 9.
Another question is how to confirm elements of the array. Therefore, there should be a for loop before the if() evaluation to have a variable loop from 0 to 11.
After certain IRcodes are confirmed, the program starts to execute the second event. There should be a corresponding executable code following every if() statement.
Code introductions are now finished. This is the most difficult section in all of the projects we have shown, and it may be difficult to understand the first time around, but take it easy - practice makes perfect.
EXERCISE
Make a DIY remote controller that works based on this project.
Using these applications, you can make a movable toy man. You could use servos, as explained in the projects mentioned before, and use different press buttons on the remote controller to have the servos move to various angles. Use your imagination and have fun.
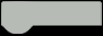