Buzzer will make different sounds by controlling the frequency and duration. What effects can you create if you can freely adjust these two variables? In this lesson, we'll build a DJ station. By using two potentiometers, you will be able to control the pitch and beat of the sound, allowing you to experience the fun of real-time sound mixing like a DJ!
COMPONENT LIST

HARDWARE
Connect the circuit according to the following wiring diagram.

fig 1 DJ Station Circuit
CODE
Sample code:
//Project - DJ Station
int pitchPin = A0; // Potentiometer 1 - analog pin A0
int beatPin = A1; // Potentiometer 2 - analog pin A1
int piezo = 8; // Piezo buzzer - digital pin 8
void setup() {
Serial.begin(9600); // Initialize the serial port at 9600 baud
}
void loop() {
int pitchVal = analogRead(pitchPin);
// Read value from pitch potentiometer
int beatVal = analogRead(beatPin);
// Read value from beat potentiometer
int frequency = map(pitchVal, 0, 1023, 0, 5000);
// Map pitch value to frequency range 0-5000 Hz
int duration = map(beatVal, 0, 1023, 100, 5000);
// Map beat value to duration range 100-5000 ms
// Print frequency and duration to the serial port
Serial.print("Frequency: ");
Serial.print(frequency);
Serial.print(" Hz, Duration: ");
Serial.print(duration);
Serial.println(" ms");
tone(piezo, frequency, duration);
// Play the tone on the piezo buzzer
int pauseBetweenNotes = duration * 1.30;
// Calculate pause between notes
delay(pauseBetweenNotes); // Wait for the pause duration
noTone(piezo); // Stop the tone
}
After successfully uploaded, open the serial monitor of Arduino IDE.
Set the baud rate 9600.

You can directly read the current frequency and duration values from the serial port. Turning the potentiometers will show changes in the readings.
CODE REVIEW
This program reads values from two potentiometers to control the frequency and duration of a tone played on a piezo buzzer. The values are also output to the serial monitor for observation.
We initialize 3 variables to assign pins for the two potentiometers and the buzzer at the top of the program.
int pitchPin = A0;
int beatPin = A1;
int piezo = 8;
In the setup, we used a new function:
Serial.begin(9600);
This function is to initialize baud rate of serial port which is data transmit speed. Normally it goes with the one in the serial monitor, except that some wireless module has specific requirement of baud rate.
In the loop function, define two new variables save the readings from two potentiometers.
int pitchVal = analogRead(pitchPin);
int beatVal = analogRead(beatPin);
Typically, variables defined at the beginning of a program are global variables, accessible and usable throughout any part of the program. In contrast, variables like pitchVal and beatVal can be defined as local variables within the loop because they are only used within the individual block of code.
There is a new function, analogRead(pin).
It reads value from analog pin. The digital pins in Arduino is connected to a 10 byte A/D converter. So the voltage between 0 and 5V is converted to a value ranging between 0 and 1023. Each value corresponds to a value of voltage.
The voltage range we read from the potentiometer is 0 to 1023. In the project[Melody Buzzer], we learned that the audible frequency range for humans is approximately 0 to 5000 Hertz (refer to project[Melody Buzzer]'s pitch file). Therefore, we need to use a new function to map the voltage range of 0 to 1023 to the frequency range of 0 to 5000 Hertz.
int frequency = map(pitchVal, 0, 1023, 0, 5000);
The format of map function is as below.
map(value, fromLow, fromHigh, toLow, toHigh)
Map function re-maps a number from one range to another. That is, a value of fromLow would get mapped to toLow, a value of fromHigh to toHigh, values in-between to values in-between, etc. It can also be used to reverse the range of values. For example: y = map(x, 1, 50, 50, 1).
Similarly, we want to use another potentiometer to control the duration of the sound, ranging from 0 seconds to 5 seconds.
int duration = map(beatVal, 0, 1023, 100, 5000);
After the serial port receive data, how to demonstrate it on the serial monitor?
Serial.print(val);
Serial.println(val);
The difference between println() and print() is that println()has a new line character.
We can use these two functions as below to output different formats of content.
Serial.print(“Frequency: ”);
Serial.print(frequency);
Although the content inside the parentheses in both statements is frequency, the first statement has double quotes around it, which outputs the content inside the quotes as a string. In the second statement, it represents the variable frequency and outputs the current value of the variable.
When outputting strings, you can add colons and spaces before and after the text content to make the output more readable.
Serial.print("Frequency: ");
Serial.print(frequency);
Serial.print(" Hz, Duration: ");
Serial.print(duration);
Serial.println(" ms");
It will looks like this on the serial monitor:

Using the same program as project[Melody Buzzer] to play sounds and set intervals. For any questions, please refer to the previous content.
HARDWARE REVIEW
Potentiometer
A potentiometer is a simple knob that provides a variable resistance ranging from 0 to 10k Ω, which we can read into the Arduino board as an analog value.
We connect three wires to the Arduino board. The first goes to ground from one of the outer pins of the potentiometer. The second goes from 5 volts to the other outer pin of the potentiometer. The third goes from analog input 2 to the middle pin of the potentiometer.

The way how potentiometer works is similar to voltage divider in project [Interact with Servo]. The potentiometer is divided into 2 resistors by the shaft. By turning the shaft of the potentiometer, we change the amount of resistence on either side of the wiper which is connected to the center pin of the potentiometer. This changes the relative "closeness" of that pin to 5 volts and ground, giving us a different analog input. When the shaft is turned all the way in one direction, there are 0 volts going to the pin, and we read 0. When the shaft is turned all the way in the other direction, there are 5 volts going to the pin and we read 1023. In between, analogRead() returns a number between 0 and 1023 that is proportional to the amount of voltage being applied to the pin.

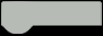