We’ve learned to turn the servo to a specific angle using an external signal. In the new project, we will use a potentiometer to control a servo. You can also modify this circuit by swapping the potentiometer for a sensor such as a tilt switch, or changing the actuator to an LED. Get tinkering and use your imagination!
COMPONENT LIST

HARDWARE
This is a little different from our previous project as we are using a potentiometer. Potentiometers are sometimes called variable resistors, and they are just that. By turning the knob, you are altering the electrical resistance of the component. It has 3 pins: two side by side and one on top. Connect the two side-by-side pins of the potentiometer to 5V and GND on the Arduino, respectively. Then, connect the single pin on the opposite side of the potentiometer to the analog 0 pin on the Arduino.

fig 1 Interact with Servo Circuit
CODE
Sample code:
// Project - Interact with Servo
#include <Servo.h> // Include the Servo library
Servo myservo; // Create a servo object
int potpin = 0; // Analog pin connected to the potentiometer
int val; // Variable to store the analog read value
void setup() {
myservo.attach(9); // Attach the servo to digital pin 9
}
void loop() {
val = analogRead(potpin);
// Read the value from the potentiometer
val = map(val, 0, 1023, 0, 179);
// Scale the value from 0-1023 to 0-179 degrees
myservo.write(val);
// Set the servo position according to the scaled value
delay(15);
// Wait for 15ms for the servo to reach the position
}
After uploading the sketch, you can see the servo turn according to the position of the potentiometer.
CODE REVIEW
We declare to insert the
Now let’s dig into the “map” function. The format of map function is as below:
map(value, fromLow, fromHigh, toLow, toHigh)
The “map” function re-maps a number from one range to another. That is, a value of “fromLow”would get mapped to “toLow”, a value of “fromHigh” to “toHigh”, values in-between to values in-between, etc.
Parameters
value: the number to map
fromLow: the lower bound of the value's current range
fromHigh: the upper bound of the value's current range
toLow: the lower bound of the value's target range
toHigh: the upper bound of the value's target range
Note that the "lower bounds" of either range may be larger or smaller than the "upper bounds" so the “map()” function may be used to reverse a range of numbers, e.g.:
y = map(x, 1, 50, 50, 1);
The function also handles negative numbers well, so that this example is also valid and works well:
y = map(x, 1, 50, 50, -100);
val = map(val, 0, 1023, 0, 179);
Back to our sketch: we map the analog value from 0 to 1023 to a servo value from 0 to 180.
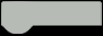