In this project, we will use the “Blink" sketch again but with a proper LED instead of the on-board LED, Through this, we can have a clear idea of how a LED works and how they can be used in a circuit.
COMPONENT LIST

*You may need to choose a different value resistor depending on the LED you will use. We will mention how to calculate resistance value in the latter part of this lesson.
HARDWARE
To build the circuit, you need to take your Arduino and stack the Prototype Shield on top of it.
The male header pins on the bottom of the Prototype Shield should line up, and slide in to the female headers on the Arduino easily. Be gentle and be careful not to bend them.
Peel the adhesive strip off the back of the Breadboard and then stick it on to the Prototype Shield. You can now set up the circuit according to the picture below.

fig 1 LED Flashing Circuit
It is standard practice to use wires of different colored insulation for your own reference, but using different combinations of colors won’t stop the circuit working.
Normally red wire indicates power supply (VCC), black wire indicates ground (GND), green wire indicates digital pins, blue wire indicates analog pins, and white is other.
Double check the orientation of LED leads on the circuit. LEDs are polarized (will only work if placed in the circuit the correct way around). The long leg of the LED connects to Vcc. (In this example Pin 13) and the short leg connects to GND, When you finish the circuit, connect the Arduino controller and computer, with the provided USB cable.
CODE
Open the Arduino IDE and enter the code as sample code shows. (We highly recommend you type code instead of copying and pasting so that you can develop your coding skills and learn to code by heart.)
Sample code:
//Project - Blinking A LED
/*
Description: turn LED on and off every other second.
*/
int ledPin = 13;
void setup() {
pinMode(ledPin, OUTPUT);
}
void loop() {
digitalWrite(ledPin, HIGH);
delay(1000);
digitalWrite(ledPin, LOW);
delay(1000);
}
When you've finished entering the code, click on "Verify" to check if the code can be compiled. If the code has no errors, click "Upload" to upload the code to the microcontroller. Now your on-board LED should be blinking on and off.
CODE REVIEW
Comments:
//Project -- Blinking A LED
Any line of code that has“//”put before it will not be compiled by the compiler. The Arduino IDE indicates this by turning the line of code gray automatically. This allows you to write plain English descriptions of the code you or others have written and makes it easier for other people who might not be able to read code to understand. We refer to this as commenting out.
Multi-Line Comments:
/ * the text between these two
symbols will be commented out;
the compiler will ignore the text
and the text will appear gray * /
Similar to single line comments, any text between / * and * / will be ignored by the compiler. Once again, the Arduino IDE will turn this text gray to show that it is commented out. This is a useful way of annotating code.
Declaring Variables:
Declaring variables is a useful way of naming and storing values for later use by the program. Integers (int) represent numbers ranging from -32768 to 32767. In the above example, we have input an integer: 13. "ledPin" is the variable name we have chosen. Of course, you may name it anything you like instead of "ledPin", but it's better to name the variable according to its function. Here, the variable ledPin indicates that the LED is connected to Pin 13 of Arduino. Use a semicolon (;) to conclude the declaration. If you don't use the semicolon, the program will not recognise the declaration, so this is important!
What is a variable?
A variable is a place to store a piece of data. It has a name, a value, and a type. In the above example, "int" (integer) is the type, "ledPin" is the name, and "13" is the value. In this example, we are declaring a variable named ledPin of type "int" (integer), meaning the LED is connected to digital pin 13. Variables display as orange text in the sketch.
Integers can store numbers from -32768 to 32767. You must introduce, or declare, variables before you use them. Later on in the program, you can simply type the variable name rather than typing out the data over and over again in each line of code, at which point its value will be looked up and used by the IDE.
When you try to name a variable, start it with a letter, followed by letters, numbers, or underscores. The language we are using (C) is case-sensitive. There are certain names that you cannot use, such as "main", "if", "while", as these have their own pre-assigned function in the IDE.
The setup() function:
The setup() function is read by the Arduino when a sketch starts. It is used it to initialize variables, pin modes, initialize libraries, etc. The setup function will only run once after each power-up or reset of the Arduino board.
In this example there is only one line in the setup() function:
pinMode(ledPin, OUTPUT);
This function is used to define the digital pin working behavior. Digital pins are defined as an input (INPUT) or an output (OUTPUT). In the example above, you can see brackets containing two parameters: the variable (ledPin) and its behavior (OUTPUT).
The function format is as follows:
pinMode configures the specified digital pin to behave either as an input or an output. It has two parameters:
pin: the number of the pin whose mode you wish to set.
mode: INPUT, OUTPUT, or INPUT_PULLUP
If you want to set the digital pin 2 to input mode, what code would you type?
Answer: pinMode(2, INPUT);
Function
Segmenting code into functions allows a programmer to create modular pieces of code that perform a defined task and then return to the area of code from which the function was "called". The typical case for creating a function is when one needs to perform the same action multiple times in a program.
There are two required functions in an Arduino sketch: setup() and loop(). Other functions must be created outside the brackets of those two functions.
Difference of INPUT and OUTPUT
INPUT is a signal sent from outside events to the Arduino, such as a button. OUTPUT is a signal sent from the Arduino to the environment, such as LED and buzzer.
If we scroll further down, we can see the main part of the code. This is the loop.
void loop() {
digitalWrite(ledPin, HIGH);
delay(1000);
digitalWrite(ledPin, LOW);
delay(1000);
}
The function format is as follows:
The Arduino program must include the setup () and loop () function, otherwise it won’t work. After creating a setup() function, which initializes and sets the initial values, the loop() function does precisely what its name suggests, and loops consecutively, allowing your program to change and respond. Use it to actively control the Arduino board. Here we want to control the LED constantly on and off every other second.
How can we make that happen?
In this project we want the LED to turn on for 1 second and then turn off for 1 second, and repeat this action over and over. How can we express this in code?
Look at the loop () function within the first statement.
This involves another function:
digitalWrite(ledPin, HIGH);
digitalWrite writes a "HIGH" (on) or a "LOW" (off) value to a digital pin. If the pin has been configured as an OUTPUT using pinMode(), its voltage will be set to the corresponding value: 5V (or 3.3V on 3.3V boards) for HIGH, and 0V for LOW. Please note that digitalWrite is applied only under the condition that pinMode is set as OUTPUT. Why? Read on!
The Relation of pinMode(), digitalWrite() and digitalRead()
If pinMode configures a digital pin to behave as an input, you should use the digitalRead() function. If the pin is configured as an output, then you should use digitalWrite().
NOTE: If you do not set the pinMode() to OUTPUT, and connect an LED to a pin, when calling digitalWrite(HIGH), the LED may appear dim. This is because without explicitly setting a pin-Mode(), digitalWrite() will enable the internal pull-up resistor, which acts like a large current-limiting resistor.

Next:
delay(1000);
delay() pauses the program for the amount of time specified (in milliseconds). (There are 1000 milliseconds in 1 second.)
HARDWARE REVIEW
Breadboard
Breadboard is a way of constructing and testing circuits, without having to permanently solder them in place. Components are pushed into the sockets on the breadboard, and then extra jumper wires are used to make connections.

fig 2 Breadboard Conductivity Direction
The breadboard has two columns, each with 17 strips of connections. In this diagram, the blue lines show how the top row is connected. This is the same for every other row. The two columns are isolated from one another.
Note that the upper and lower parts of the ravine are not connected. and this ravine is a standard spacing and perfect for DIP ICs fitting into breadboards as shown in the following figure.

fig 3 DIP IC Inserted
Resistors
Resistors, as the name suggests, resist the flow of electricity. The higher the value of the resistor, the more resistance it has, and the less electrical current will flow through it. The unit of resistance is called the Ohm, which is usually shortened to Ω (the Greek letter Omega). Unlike LEDs, resistors are not polarized (do not have a positive and negative lead) - they can be connected either way around. Normally, a LED needs 2V of voltage and 35mA of current to be lit, so with a resistor of 100Ω, you would be able to control the flow of electricity. If less than 100Ω, you might risk burning it out. Be careful of this, because resistors can get hot!
Read Resistor Color Rings
The resistor value will be marked on the on the outer package of your resistors, but what should we do in case the label gets lost and there are no measuring tools at hand? The answer is to read the resistor value. This is a group of colored rings around the resistor. Details are available online for those who are interested in having a try.
Here is an online calculator for resistor value calculating:
https://www.omnicalculator.com/physics/resistor-color-code(If the link fails, use the resistance calculator as a keyword to search for available web pages or software.)
LEDs
A light-emitting diode (LED) is a two-lead semiconductor light source. Typically, LEDs have two leads: one positive and one negative. There are two ways to determine which lead is positive and which is negative: Firstly, the positive lead is typically longer. Secondly, where the negative lead enters the body of the LED, there is a flat edge on the LED's casing.
LEDs are polarized, meaning they must be connected in the correct orientation. If you connect the negative lead of an LED to the power supply and the positive lead to ground, the LED will not function, as shown in the diagram.

In your kit, you can also find LEDs with four leads. These are RGB LEDs that contain three primary color LEDs embedded within them. We will explore this further later.
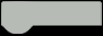