This project aims to achieve a dice rolling function through Arduino and digital tube. When the tilt switch is triggered, the digital tube will randomly display a number between 0 and 9, simulating the effect of rolling a dice.
COMPONENT LIST
HARDWARE

fig 1 Digital Dice Roller Circuit
CODE
Sample code:
// Project - Digital Dice Roller
// Define pins for the digital tube
const int pinA = 3;
const int pinB = 2;
const int pinC = 8;
const int pinDP = 9;
const int pinF = 4;
const int pinG = 5;
const int pinE = 6;
const int pinD = 7;
// Define pin for the tilt switch
const int tiltSwitchPin = 13;
int sensorValue;
int lastTiltState = HIGH;
// Previous state read from the tilt sensor
unsigned long lastDebounceTime = 0; // Last time checked
unsigned long debounceDelay = 500;
// Debounce delay time in milliseconds
/* 2D array defining the display patterns for numbers 0-9 on the digital tube */
int numbers[10][8] = {
{0, 0, 0, 0, 0, 0, 1, 1}, // Number 0
{1, 0, 0, 1, 1, 1, 1, 1}, // Number 1
{0, 0, 1, 0, 0, 1, 0, 1}, // Number 2
{0, 0, 0, 0, 1, 1, 0, 1}, // Number 3
{1, 0, 0, 1, 1, 0, 0, 1}, // Number 4
{0, 1, 0, 0, 1, 0, 0, 1}, // Number 5
{0, 1, 0, 0, 0, 0, 0, 1}, // Number 6
{0, 0, 0, 1, 1, 1, 1, 1}, // Number 7
{0, 0, 0, 0, 0, 0, 0, 1}, // Number 8
{0, 0, 0, 0, 1, 0, 0, 1} // Number 9
};
void setup() {
Serial.begin(9600);
// Set pins for the digital tube as OUTPUT
pinMode(pinA, OUTPUT);
pinMode(pinB, OUTPUT);
pinMode(pinC, OUTPUT);
pinMode(pinDP, OUTPUT);
pinMode(pinF, OUTPUT);
pinMode(pinG, OUTPUT);
pinMode(pinE, OUTPUT);
pinMode(pinD, OUTPUT);
// Set tilt switch pin as INPUT with internal pull-up resistor
pinMode(tiltSwitchPin, INPUT_PULLUP);
}
void loop() {
unsigned long currentTime = millis();
int sensorValue = digitalRead(tiltSwitchPin);
// If the tilt switch state changes and debounce delay has passed:
if (sensorValue != lastTiltState && (currentTime - lastDebounceTime) > debounceDelay) {
int randomNumber = random(0, 10);
// Generate a random number from 0 to 9
displayNumber(randomNumber); // Display the random number
// Update state variables and debounce time:
lastTiltState = sensorValue;
lastDebounceTime = currentTime;
}
}
// Display the number on the digital tube
void displayNumber(int number) {
digitalWrite(pinA, numbers[number][0]);
digitalWrite(pinB, numbers[number][1]);
digitalWrite(pinC, numbers[number][2]);
digitalWrite(pinD, numbers[number][3]);
digitalWrite(pinE, numbers[number][4]);
digitalWrite(pinF, numbers[number][5]);
digitalWrite(pinG, numbers[number][6]);
digitalWrite(pinDP, numbers[number][7]);
// Change this to 0 if you need to display the decimal point
}
CODE REVIEW
Outside the setup() function, the variable definitions might be familiar to you, and are best understood by reviewing the code comments. To define the pins for the digital tube, please refer to the project[Infrared Controlled LED Matrix].
Within the setup() function, you will set the pins for the digital tube as outputs, and configure the tilt switch pin as an input with an internal pull-up resistor enabled, as follows:
pinMode(tiltSwitchPin, INPUT_PULLUP);
When not triggered, the pin reads as high (HIGH).
Continuing with the loop() function, a crucial aspect is implementing software debouncing for the tilt switch.
Continuing with the loop() function, a crucial aspect is implementing software debouncing for the tilt switch through programming.
The logic behind debouncing involves comparing the current state with the previous state, and checking if the time interval between state changes exceeds a predefined debounce delay time. This approach prevents multiple triggerings caused by the mechanical bouncing of the contacts inside the physical switch when it is activated or deactivated.
First, define the variable lastDebounceTime, which is used to store the timestamp of the last detected change in the tilt switch state. debounceDelay is set to 500 milliseconds for this project. This implies that if the state of the tilt switch changes frequently within 500 milliseconds, these changes will be considered as bounces and will not be treated as valid state changes.
unsigned long lastDebounceTime = 0;
unsigned long debounceDelay = 500;
Inside the loop() function, first obtain the current timestamp (currentTime) and the current state value of the tilt switch (sensorValue).
unsigned long currentTime = millis();
int sensorValue = digitalRead(tiltSwitchPin);
Afterwards, the program will check the state of the tilt switch (sensorValue). If the current state (sensorValue) is different from the last state (lastTiltState), and the time interval exceeds the set debounce delay time, then the program will consider this as a valid state change.
if (sensorValue!=lastTiltState && (currentTime-lastDebounceTime) > debounceDelay){
......
}
If a valid change is detected, then perform the operations within the if statement:
int randomNumber = random(0, 10);
displayNumber(randomNumber);
lastTiltState = sensorValue;
lastDebounceTime = currentTime;
Generate a random number, and call the displayNumber() function to display the random number on the digital display. Then, update the state variables and reset the debounce time.
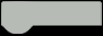