In this project, we will create a drum pad that explores how Arduino captures the signals generated by tapping and controls a buzzer to produce sounds of different frequencies. By varying the pressure applied, you will experience the real-time change in sound frequency, feeling the magic and joy of the electronic world.
COMPONENT LIST

HARDWARE
We have connected buzzers many times before, and all we need to do now is add the relevant circuitry for the piezo disk. The piezo disk circuit also includes a 1M resistor and a 5.1V Zener diode. Please note the direction of the Zener diode connection (as shown in Fig 1).

fig 1 Drum Pad Circuit
CODE
Sample code:
// Project - Drum Pad
// These constants do not change. They are used to name the pins used:
const int analogInPin = A0; // Analog input pin, piezo diskconst int analogOutPin = 8; // Analog output pin, buzzer
int sensorValue = 0; // Value read from the piezo disk
int outputValue = 0; // Value output to PWM (analog output)
void setup() {
// Initialize serial communication with a baud rate of 9600:
Serial.begin(9600);
}
void loop() {
// Read the analog input value:
sensorValue = analogRead(analogInPin);
// Map it to the range of the analog output:
outputValue = map(sensorValue, 0, 1023, 0, 5000);
/* Change the analog output value (note: Arduino pin 8 is digital, using tone function to simulate sound) */
tone(analogOutPin, outputValue, 100);
/* Use the tone function to generate sound on pin 8 (digital output) with frequency outputValue and duration 100ms */
// Print the result to the serial monitor:
Serial.print("sensor=");
Serial.println(sensorValue);
}
When the piezo disk is struck, the buzzer will emit sounds with varying pitches. Simultaneously, observe your serial monitor, where the "sensor" value will quickly climb to its maximum and then drop back to 0. Different values reflect the intensity of the pressure or strike on the piezo disk, with higher values indicating more vigorous impacts. If nothing is observed, please check your connections, especially the direction of the piezo disk and Zener diode.
CODE REVIEW
At the beginning of the code, we encounter a new data type, const, which we haven't used before. In previous projects, we encountered several common data types (like int, long, etc.). In this project, we introduce a new data type: const, which stands for constant.
const int analogInPin = A0;
const int analogOutPin = 8;
int sensorValue = 0;
int outputValue = 0;
In the above code, analogInPin and analogOutPin are defined as constants, representing the analog input pin connected to the piezo disk and the analog output pin connected to the buzzer, respectively.
Next, two integer variables, sensorValue and outputValue, are declared. The former stores the raw analog value read from the piezo disk, while the latter stores the mapped value (converted to a range that the buzzer can produce sounds).
Next, initializing the setup() function is quite straightforward, simply requiring the setting of a serial port baud rate, which enables us to monitor and debug the input signals for the piezo disk.
In the main loop(), the analog signal generated by the piezo disk is read and stored in sensorValue.
Next, the sensorValue is mapped from a range of 0 to 1023 to a range of 0 to 5000 using the following statement:
outputValue = map(sensorValue, 0, 1023, 0, 5000);
This is because the output range of the piezo disk is typically 0 to 1023, whereas the frequency parameter of the tone() function needs to be within the frequency range that Arduino can generate (typically 31Hz to 65535Hz, though actual performance is limited by hardware).
tone(analogOutPin, outputValue, 100);
Generates a sound with a frequency of outputValue (controlled by the piezo disk) on the analogOutPin pin for a duration of 100 milliseconds. The tone() function, based on PWM (Pulse Width Modulation) technology, drives the buzzer to emit sounds of different frequencies. PWM technology enables digital pins to output the effect of analog signals by rapidly switching the digital signal between high and low levels (i.e., on and off) at a specific rate, simulating signals of different frequencies. For more information on PWM technology, refer to the project[Fading Light].
Serial.print("sensor=");
Serial.println(sensorValue);
Finally, to facilitate program debugging and observe the real-time value of the piezo disk, prints the value of sensorValue.
HARDWARE REVIEW
Piezo Disk
Piezo disk, also known as piezoelectric transducers, derive from the term "piezoelectric," meaning "current generated by pressure." When a piezoelectric device is compressed, it produces an electric charge, as shown in Fig 2. A typical application of transducers with Arduino is as a striking sensor. When the transducer is hit or impacted, Arduino can detect it and trigger corresponding actions, in this project, generating sounds related to the striking force.
Conversely, when an electric charge is applied to a piezoelectric transducer, it deforms, as shown in Figure 3. If a voltage varying at a certain frequency is applied to it, the transducer's vibrations produce sound or melodies.

fig 2 When the piezoelectric transducer is deformed, it will generate a charge. Squeezing and releasing the transducer will generate a transformed voltage.

fig 3 When a changing voltage is applied to the piezoelectric transducer, the transducer will be deformed.
Figures 2 and 3 illustrate that a piezoelectric transducer can function as both an input device and an output device. It converts striking signals into electrical signals and electrical signals into vibration frequencies.
When using piezo disk, special attention should be paid to its polarity, which is distinguished by a red and a black line welded with low-melting solder. The black line connects to the GND part of the circuit, and the red line connects to the analog input pin of Arduino.
Zener Diode
In the project[Blinking A LED], we introduced Light-Emitting Diodes (LEDs). The project also features a Zener diode, a special type of diode designed to allow current to flow in reverse when the voltage exceeds its breakdown voltage (or knee voltage).
To protect the Arduino's analog input pins from exceeding 5V, the Zener diode must be connected correctly. Conventionally, the cathode or negative terminal of the diode, often marked with a black stripe, is connected to GND. However, in this circuit, you'll reverse-bias the diode, meaning the cathode is connected to the circuit's positive voltage source. The Zener diode conducts only when the reverse voltage exceeds its breakdown voltage, which in this case is 5.1V. Any voltage above 5.1V will cause the diode to conduct in reverse, effectively shorting to GND and protecting the Arduino's input pins.
EXERCISE
In our hardware review, we learned that piezo disk can function both as an input device and an output device. Try using the tone() function to write signals to the piezo disk, making it emit a specified frequency (in Hz). Combine these frequencies to create a melody! (Note: The sound emitted by piezo disk is much softer than that of a buzzer, so please listen carefully!)
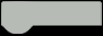