Let’s start with a new component: an RGB LED. This component combines red, blue and green LEDs and can display various colors by adjusting the different values of each light. A computer monitor uses many RGB LEDs to display an image. In this lesson, We will learn how to create random color with an RGB LED.
COMPONENT LIST
HARDWARE

fig 1 RGB LED Circuit
To connect your RGB LED (common cathode type in this kit), refer to Fig. 1. Unsure if a RGB LED which does not from this kit is a common cathode or common anode? Check the HARDWARE REVIEW section for clarification.
CODE
Sample code:
// Project - RGB LED
int redPin = 9;
int greenPin = 10;
int bluePin = 11;
void setup(){
pinMode(redPin, OUTPUT); // Set the red pin as an OUTPUT
pinMode(greenPin, OUTPUT); // Set the green pin as an OUTPUT
pinMode(bluePin, OUTPUT); // Set the blue pin as an OUTPUT
}
void loop(){
colorRGB(random(0,255),random(0,255),random(0,255));
// Generate random RGB values (R:0-255, G:0-255, B:0-255)
delay(1000); // Wait for 1 second
}
void colorRGB(int red, int green, int blue){
analogWrite(redPin,constrain(red,0,255));
/* Write the red value to the red pin, constraining it between 0 and 255 */
analogWrite(greenPin,constrain(green,0,255));
/* Write the green value to the green pin, constraining it between 0 and 255 */
analogWrite(bluePin,constrain(blue,0,255));
/* Write the blue value to the blue pin, constraining it between 0 and 255 */
}
After uploading this code, You should see the RGB LED blinking with random colors.
CODE REVIEW
First, we will configure the 3 LEDs contained within the RGB LED to 3 PWM pins so we can adjust them to different colors by setting 3 pins as an OUTPUT.
The main part is to create a function called colorRGB() that takes three parameters: the red, green, and blue values, which range from 0 to 255. With this function, we can easily specify colors for RGB LEDs without having to repeatedly write the analogWrite() command. This way, when you need to set colors, you just call colorRGB() and pass in the RGB value.
Here we will introduce constrain() and random().
Try to look them up with the websites we mentioned in the project's[Fading Light] homework first and see if you can understand them.
The format of the constrain function is as follows:
The constrain() function requires three parameters:
x, a and b.
x is a constraint number here, a is the minimum, and b is the maximum.
If the value is less than a, it will return to a. If it is greater than b, it will return to b.
Red, green and blue are our constrained parameters. They are constrained between 0 and 255 (which falls into the range of PWM values). Values are generated at random using the random() function.
The format of random() is as below:
The first variable of this function is the minimum value and the second is the maximum. So we configure it as random(0,255) in this program.
HARDWARE REVIEW
RGB LED
The RGB LED has four leads. If you are using a common cathode RGB LED, there is one lead going to the positive connection of each of the single LEDs and a single lead that is connected to all three negative sides of the LEDs. That’s why it is called the common cathode. There is no difference in appearance between common cathode and common anode RGB LEDs, however, you do need to pay attention when assigning color values. For example, for the common cathode RGB LED, red is "R-255, G-0, B-0". For the common anode RGB LED, red is R-0, G-255, B-255.
How can we adjust the RGB LED to change to different colors?
By assigning different values of brightness levels to 3 primary colors using the function analogWrite(value), you can configure any color you like!

fig 2 How 3 LEDs form A RGB LED (Common Cathode)

fig 3 Remixing red, green and blue to achieve various colors
You can configure 255x255x255(16777216) kinds of colors by assigning different PWM values on these 3 LEDs!

form 1 Colors generated from combined PWM values of different LEDs
The difference between common anode and common cathode RGB LEDs
What is the difference between common anode and common cathode, RGB LEDs in application? According to the figure below, there is no difference between common anode and common cathode in terms of their appearance. However, there are two key differences in their application:
(1) Different connections: for the common anode, the common port should be connected to 5V but not GND, otherwise the LED fails to be lit.
(2) Color matching: For a common cathode RGB display, displaying red would be R-255, G-0, B-0. However, for a common anode, it's exactly the opposite, with RGB values being R-0, G-255, B-255.

fig 4 Common Anode RGB Diagram

fig 5 Common Cathode RGB Diagram
How to recognize an RGB LED is a Common Anode or a Common Cathode?
Actually, it’s very simple and doesn’t even require programming to verify. RGB LEDs have separate LEDs for red, green, and blue. In a common cathode configuration, the common pin is connected to ground, requiring a positive voltage on the R, G, and B pins to light up. In a common anode configuration, the common pin is connected to the positive voltage, requiring the R, G, and B pins to be connected to ground for lighting.
The circuit diagrams for common cathode and common anode configurations are as follows:

fig 6 Circuit Diagram for Common Cathode and Common Anode
The test method is as follows:
1.Connect a 220Ω resistor to each of the R, G, and B pins.
2.For testing, first connect the common pin to ground, then connect each R, G, and B pin to the positive voltage one at a time. If the correct color lights up, it’s a common cathode. If not, it’s likely a common anode.
3.To confirm, repeat the test with the opposite configuration (common pin to positive and R, G, B pins to ground) to ensure that the failure to light up isn’t due to a faulty RGB LED.
EXERCISE
1. Based on the program above, create a color changing rainbow sequence. Try to configure various colors by, playing with different values. TIP: You only need to change the variables of "colorRGB".
2. Take your rainbow sequence LED and make each color fade so that the transition between each color is smoother.
3. Trying to load a pre-packaged rgbled library in the Arduino IDE and utilizing existing functions within it can help achieve more functionalities. Below is a case that demonstrates how to add a library file and use that library.

fig 7 Tools->Mange Libraries

fig 8 Searching "rgbled"->INSTALL

fig 9 rgbled installed auccessfully

fig 10 File->Examples->rgbled

fig 11 Verify and Upload
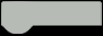