In daily life, LED matrix display is a commonly used device that can present information in a matrix form, such as numbers, letters, or simple graphics. By controlling the on and off states of each dot (LED) on the dot matrix display, we can create various visual effects. Today, we will learn how to control an 8*8 LED matrix display through an Arduino project, including how to initialize the hardware, write code to light up all the LEDs, and implement scanning display technology.
COMPONENT LIST
HARDWARE
fig 1 Light Up Matrix Circuit
CODE
Sample Code:
//Project - Light Up Matrix
const int rowPins[8] = {6, 11, 5, 9, A0, 4, A1, 2}; // Row pins for the dot matrix display (negative)
const int colPins[8] = {10, A2, A3, 7, 3, 8, 12, 13}; // Column pins for the dot matrix display (positive)
void setup() {
for (int i = 0; i < 8; i++) {
pinMode(colPins[i], OUTPUT);
pinMode(rowPins[i], OUTPUT);
digitalWrite(rowPins[i], HIGH);
// Initialize row pins to high level (off)
}
}
void turnOnAllLeds() {
for (int i = 0; i < 8; i++) {
digitalWrite(colPins[i], HIGH);
digitalWrite(rowPins[i], LOW);
}
}
void turnOffAllLeds() {
for (int i = 0; i < 8; i++) {
digitalWrite(colPins[i], LOW);
digitalWrite(rowPins[i], HIGH);
}
}
void scanRows() {
digitalWrite(colPins[0], HIGH);
for (int col = 0; col < 8; col++) {
digitalWrite(rowPins[col], LOW);
delay(500);
}
turnOffAllLeds();
}
void scanColumns() {
for(int col = 0; col < 8; col++){
digitalWrite(colPins[col], HIGH);
for(int row = 0; row < 8; row++){
digitalWrite(rowPins[row], LOW);
}
delay(500);
digitalWrite(colPins[col], LOW);
}
}
void loop() {
turnOnAllLeds();
delay(1500);
turnOffAllLeds();
delay(1500);
scanRows();
scanColumns();
}
After successfully uploading the program, you can observe the LED matrix display flash once, followed by the LEDs in the first column lighting up sequentially from top to bottom. Subsequently, the LEDs in each column of the LED matrix will light up in sequence from left to right, and these effects will repeat continuously.
CODE REVIEW
Before you start learning how to control the 8*8 LED matrix screen using code, it is recommended that you review the hardware view section to understand the basic principles of the 8*8 LED matrix screen. This will make it easier for you to comprehend and get started with the dot matrix screen lighting effects demonstrated in the code of this project.
const int rowPins[8] = {6, 11, 5, 9, A0, 4, A1, 2};
const int colPins[8] = {10, A2, A3, 7, 3, 8, 12, 13};
The initial part of this code defines two arrays of pins, rowPins and colPins, which are used to connect to the rows and columns of the LED matrix display respectively.
void setup() {
for (int i = 0; i < 8; i++) {
pinMode(colPins[i], OUTPUT);
pinMode(rowPins[i], OUTPUT);
digitalWrite(rowPins[i], HIGH);
}
}
The setup() function executes solely once upon powering up the Arduino board.
By employing a for loop, the pins contained within the colPins and rowPins arrays are configured in output mode.
Additionally, all row pins are set to HIGH to guarantee that the dot matrix display remains in an inactive state during the initialization process.
void turnOnAllLeds() {
for (int i = 0; i < 8; i++) {
digitalWrite(colPins[i], HIGH);
digitalWrite(rowPins[i], LOW);
}
}
The turnOnAllLeds() function uses a for loop to set all column pins to high level (HIGH) and all row pins to low level (LOW), resulting in all LED lights being turned on.
void turnOffAllLeds() {
for (int i = 0; i < 8; i++) {
digitalWrite(colPins[i], LOW);
digitalWrite(rowPins[i], HIGH);
}
}
The turnOffAllLeds() function turns off all LEDs on the LED matrix display by setting all column pins to LOW and all row pins to HIGH.
void scanRows() {
digitalWrite(colPins[0], HIGH);
for (int row = 0; row < 8; row++) {
digitalWrite(rowPins[row], LOW);
delay(500);
}
turnOffAllLeds();
}
The scanRows() function scans the rows, lighting up the LEDs in the first column sequentially from the first row to the eighth row.
void scanColumns() {
for(int col = 0; col < 8; col++){
digitalWrite(colPins[col], HIGH);
for(int row = 0; row < 8; row++){
digitalWrite(rowPins[row], LOW);
}
delay(500);
digitalWrite(colPins[col], LOW);
}
}
The scanColumns() function scans the columns. It uses two nested for loops: the outer loop iterates through all the columns, and the inner loop lights up all the LEDs for the current column.
void loop() {
turnOnAllLeds();
delay(1500);
turnOffAllLeds();
delay(1500);
scanRows();
scanColumns();
}
The loop() function is the main body of the Arduino program's loop.
It attempts to turn on all LEDs, waits for a delay of 1.5 seconds, then turns off all LEDs and waits for another 1.5-second delay.
Subsequently, it calls the scanRows() and scanColumns() functions to achieve the effect of sequentially scanning rows and columns.
HARDWARE REVIEW
8*8 LED Matrix
The dot matrix display used in this project is of the 788AS specification, with an internal circuit diagram shown in Figure 2. From Figure 2, it can be seen that the LED display is an 8x8 dot matrix display composed of 64 light-emitting diodes (LEDs). Each LED is placed at the intersection of a row line and a column line. When a specific column is set to high level and a specific row is set to low level, the corresponding LED will light up.
fig 2 internal Circuit Diagram
EXERCISE
In this project, rows are scanned before columns. Please try to modify the program to achieve column scanning before row scanning.
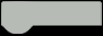