Let’s try a new component: the buzzer! It generates sounds of different frequencies using sinusoidal waves. If you connect an LED with the same sinusoidal wave, you can make yourself an alarm.
COMPONENT LIST
HARDWARE
Make the following connections. Please note that the buzzer of this project is a passive buzzer without positive and negative terminals. The two pins of the buzzer are connected to GND and digital port 8 respectively.

fig 1 Alarm circuit
CODE
Sample code:
// Project - Alarm
float sinVal;
int toneVal;
void setup(){
pinMode(8, OUTPUT); // Set pin 8 as an output pin
}
void loop(){
for(int x=0; x<180; x++){
// Convert sin function angle to radians
sinVal = (sin(x*(3.1412/180)));
// Calculate tone frequency based on sin value
toneVal = 2000+(int(sinVal*1000));
// Generate sound frequency using the sin function value
tone(8, toneVal); // Output toneVal to pin 8
delay(2); // Pause for 2 milliseconds
}
}
You can hear the alarm of high and low pitches after uploading the code.
CODE REVIEW
First, define two variables:
float sinVal;
int toneVal;
“float” is a datatype for floating point numbers (a number that has a decimal point).
Floating point numbers are often used to approximate analog and continuous values because they have greater resolution than integers. Here we use the “float” variable to store sinusoidal values. The sinusoidal wave changes quite evenly in a wave shape(Just like the wave form in fig 2), so we convert it to sound frequencies. Hence, toneVal gets values from sinVal and converts them to frequencies.

fig 2 sin()
Since the frequency value cannot be negative, we choose the sine function within the radian range of 0 to π, which corresponds to the angle range of 0 to 180 degrees.
for(int x=0; x<180; x++)
{
}
We need to use the formula (3.1412/180) to convert it from an angle to a radian value because the unit “sin” is radian instead of an angle.
sinVal = (sin(x*(3.1412/180)));
Then we change this value to a sound frequency of an alarm:
toneVal = 2000+(int(sinVal*1000));
“sinVal” is a floating variable, a value with a decimal point. We don’t want our frequency to have a decimal point, so we need to change the floating value to an integer value by writing the command as below:
int(sinVal*1000)
Human ears can notice sound in frequencies from 20Hz to 20kHz, so we multiply the raw value by 1000 times plus 2000 to assign the value for “toneVal” to give us a range of 2000 to 3000.
tone(8, toneVal);
Here we introduce 3 functions relevant to tone:
1. tone(pin, frequency)
“pin” is the digital pin connected to the buzzer. Frequency is the frequency value in Hz.
2. tone(pin, frequency, duration)
The “duration” parameter is measured in milliseconds. If there is no duration, the buzzer will keep making sounds of different frequencies.
3. noTone(pin)
The “noTone(pin)” function is to end the sound from the specific pin.
HARDWARE REVIEW
The Buzzer
A buzzer is an electronic component that can generate sound. There are generally two types: piezo buzzers and magnetic buzzers.
The Difference between Active Buzzers and Passive Buzzers
Piezo and magnetic buzzers are further categorized into two types: active and passive buzzers. The basic difference lies in different demands for their input signal. In this case, “active” and “passive” do not refer to power sources, but rather to oscillation sources.
An active buzzer has its own oscillation source - it buzzes when it is powered on.
An active buzzer has a simple oscillator circuit that changes DC current into a pulse signal of a certain frequency. Active buzzers contain a special film called “molybdenum”. The magnetic field from the oscillation of the buzzer. Once powered, it starts to make a sound.
Active buzzers are polarized, so they have to be connected correctly: They have a long lead (anode) and short lead (cathode)
In this kit, passive buzzers are included.
A passive buzzer has no oscillator of its own, so it needs to use a square wave from 2khz to 5khz to trigger it instead of simply using direct current.
Passive buzzers are non-polarized meaning that they will work regardless of the direction of the current flow.
If you want to explore buzzers further, here are some project ideas:
Passive buzzers are good for various musical effects.
There are many applications based on buzzers. A lot of buzzer-based gadgets are possible like infrared sensors and ultrasonic sensors for monitoring and alerting approaching objects; temperature sensor for excess temperature alarm; and gas sensors for gas leakage alarms. Besides alarms, buzzers can also be used as musical instruments using different frequencies to form different notes.
Aren’t buzzers amazing?
EXERCISE
1. Make an alarm with a red LED.
Set up our circuit so that the LED changes in unison with the “sin” function so that the light intensity changes with the sound.
2. Using what you learned in Project[Traffic Light], can you make a doorbell? When the button is pressed, the buzzer should make a sound.
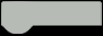