Let’s introduce a new sensor component: the photo diode. In simple terms, when the sensor detects light, its resistance changes. The stronger the light in the surrounding environment, the lower the resistance value the photo diode will read. By reading the photo diode’s resistance value, we can work out the ambient lighting in an environment. The photo diode provided in the starter kit is a typical light sensor.
In this project, we will make an automatic light that can adjust itself according to the ambient lighting around it. When it is dark, the photo diode detects the change and triggers the light, and vice versa.
COMPONENT LIST

HARDWARE
Be aware that photo diodes are polarized, just like LEDs, so they will only work if connected the correct way around.
The photo diode has to be connected with a 10k resistor rather than a 220Ω resistor.

fig 1 Auto Light Circuit
CODE
Sample code:
// Project - Auto Light
int LED = 13; // Define LED pin as digital pin 13
int val = 0;
// Define variable to store analog reading from pin 0 (light sensor)
void setup(){
pinMode(LED, OUTPUT); // Set the LED pin as OUTPUT mode
Serial.begin(9600);
// Initialize serial communication with a baud rate of 9600
}
void loop(){
val = analogRead(0);
// Read analog value from pin 0 (ranging from 0 to 1023)
Serial.println(val);
// Print the analog value to the serial monitor
if(val < 1000){
// If the analog value is less than 1000,
digitalWrite(LED, LOW); // turn the LED OFF
}else{ // Otherwise,
digitalWrite(LED, HIGH);// turn the LED ON
}
delay(10);
// Wait for 10 milliseconds before the next loop iteration
}
After uploading the code, you can shine a flashlight on the photo diode to alter the light levels in the environment. When it is dark, it should light up. When it is bright, the LED should turn off.
CODE REVIEW
A very brief explanation of the program:
Similar to the LM35 temperature sensor, the photo diode reads an analog signal so we don’t need to define “pinMode” in “serial.begin”.
We take the analog current data from the photodiode and compare it to a value of 1024 to make it digital. You can change this value if you like. Try playing with the serial monitor and seeing what outputs the photodiode gives. Then use the number you get here as the comparison number to alter the sensitivity of the circuit.
HARDWARE REVIEW
Photo Diode
A photo diode is a semiconductor device that converts light into current. The current is generated when photons are absorbed in the photo diode. The stronger the environment’s light, the lower the resistance value the photo diode will output. The analog value ranging from 0 to 1023 corresponds to voltage value ranging from 0 to 5V.
The input voltage Vin(5V) is connected to 2 resistors. By measuring the voltage of R2 as below, you can get the resistance value of photo diode.
In our project, R1 is the 10k resistor and R2 is the photo diode. The resistance value of R2 in the dark is so high that it almost reaches 5V. Once photons are absorbed, the value of R2 will decrease, and so will its voltage value. For this project it is preferable to use a fixed resistor ranging from 1k to 10k, otherwise the voltage dividing ratio is not obvious. This is why in this project we have used a 10k resistor for R1.

fig 2 Diagram of voltage divider

fig 3 Formula of voltage divider
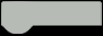