In this project, we have created a fingertip-controlled switching device using LED lights, transistors, and resistors. Simply by lightly touching with our fingertips, the LED light instantly turns on, showcasing the amazing interaction of electronic components. This project is both simple and fascinating, allowing us to explore the mysteries of the electronic world through hands-on experience.
COMPONENT LIST
HARDWARE
Note that this project utilizes two resistors with different resistance values. Ensure proper distinction and connection of these resistors during assembly. Make sure all components are connected correctly, avoiding polarity errors or reversed pins. Please refer to Fig 1 for guidance on connections.
fig 1 Touch Switch Circuit
CODE
Sample code:
int ledPin = 13;
int NPNPin = 0;
void setup()
{
pinMode(ledPin, OUTPUT); // Set the digital pin as OUTPUT
Serial.begin(9600);
// Initialize serial communication with a baud rate of 9600
}
void loop()
{
int n = analogRead(NPNPin);
// Read analog value from the NPN transistor pin
Serial.println(n);
// Print the read value to the serial monitor
if (n > 0)
// If there is a voltage response (above 0)
{
digitalWrite(ledPin, HIGH); // Turn the LED on
delay(100);
// Delay for 100 milliseconds to make the LED visible longer
digitalWrite(ledPin, LOW); // Turn the LED off
}
}
After uploading the code and connecting the two jumper wires with your fingers, you will notice the LED light up. When you separate the two jumper wires, the LED will turn off.
CODE REVIEW
The code for this project is relatively easy to comprehend, without involving any new statements or concepts. The setup() function performs two routine operations: setting the pin modes and initializing serial communication.
Within the loop() function, the statement int n = analogRead(NPNPin); reads an analog value from analog pin 0 and stores it in the variable n. The range of analog signals is typically from 0 to 1023. This read analog signal is then sent to the computer via serial communication and displayed in the serial monitor.
if (n > 0) // If there is a voltage response (above 0)
{
digitalWrite(ledPin, HIGH); // Turn the LED on
delay(100);
// Delay for 100 milliseconds to make the LED visible longer
digitalWrite(ledPin, LOW); // Turn the LED off
}
The if(n > 0) statement checks if the value of the variable n (the analog signal output by the NPN transistor) is greater than 0. If true, it indicates that the NPN transistor is outputting an analog signal greater than zero.
Inside the If statement, the digitalWrite(ledPin, HIGH) statement outputs a high voltage level to the digital pin connected to the LED, turning on the LED light. Following this, the delay(100); statement pauses the program execution for 100 milliseconds, allowing the LED to remain lit for a brief period.
Afterward, the digitalWrite(ledPin, LOW) statement sets the digital pin connected to the LED to a low voltage level, turning off the LED light.
By connecting our bodies to the circuit, we utilize the human body as an external resistor, enabling us to "truly" interact with the circuit by turning the LED light on and off.
HARDWARE REVIEW
NPN Transistor
The NPN transistor, forming three terminals: the base (B), the collector (C), and the emitter (E) (as shown in fig 2). We can simplify the transistor as a switch: when there is no current or minimal current at the B terminal, the CE can be considered in an off-state(not conducting); when current flows into the B terminal, the CE is considered in an on-state, and at this point, the size of the emitter current (IE) has a proportional relationship with the current flowing into the B terminal, determined by the transistor's amplification factor.
The variation in emitter current can indirectly reflect the change in the intensity of the output analog signal, and the amplification effect of the transistor enables this change to be amplified and output as a usable analog signal voltage.
fig 2 NPN transistor
In this project, by connecting a resistor to the Base (B) and establishing a connection, we effectively provide the Base with a small current, enabling the transistor to conduct, allowing current to flow out of the Emitter (E). According to Ohm's Law, which states U=IR, where voltage (U) is constant, the current (I) decreases as the resistance (R) in series with the base increases. Therefore, when reading the serial port values, it will be observed that a larger resistance in series with the base (such as the human body, with a resistance ranging from 1MΩ to 6MΩ) results in a smaller analog signal being read. Conversely, if the two ends of the wires are directly connected, a larger analog signal will be read.
When the Base (B) is not connected or is open, the Base current (IB) is zero, resulting in both the Collector current (IC) and the Emitter current (IE) being zero as well.
In this project, the workflow involving the NPN transistor is as follows:
1. Input: read the analog signal from NPN's Emitter.
2. processing: comparison.
3. Output: turning on or off the LED light.
EXERCISE
Based on the principle that the circuit of this project can reflect the resistance value of the resistor connected in the circuit by reading the analog signal from an NPN transistor, we aim to create a resistance detection device that utilizes an LED light to visually indicate the size of the resistance. Specifically, we desire that as the resistance value of the connected resistor increases, the LED light becomes brighter; conversely, when the resistance value decreases, the LED light dims.
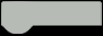