In Project[RGB LED], we learned about how to adjust an RBG LED to various colors. This time we will try to make it interactive by adding 3 potentiometers so you can choose any color you want for your lighting at home.
COMPONENT LIST

HARDWARE

fig 1 RGB Light Dimmer Circuit
CODE
Sample code:
CODE
// Project - RGB Light Dimmer
int redPin = 9; // R - digital pin 9
int greenPin = 10; // G - digital pin 10
int bluePin = 11; // B - digital pin 11
int potRedPin = 0; // Potentiometer 1 - analog pin 0
int potGreenPin = 1; // Potentiometer 2 - analog pin 1
int potBluePin = 2; // Potentiometer 3 - analog pin 2
void setup(){
pinMode(redPin, OUTPUT);
pinMode(greenPin, OUTPUT);
pinMode(bluePin, OUTPUT);
Serial.begin(9600); // Initialize serial communication
}
void loop(){
int potRed = analogRead(potRedPin);
// potRed stores the value read from analog pin 0
int potGreen = analogRead(potGreenPin);
// potGreen stores the value read from analog pin 1
int potBlue = analogRead(potBluePin);
// potBlue stores the value read from analog pin 2
int val1 = map(potRed, 0, 1023, 0, 255);
// Convert potRed value to 0-255 range using map function
int val2 = map(potGreen, 0, 1023, 0, 255);
int val3 = map(potBlue, 0, 1023, 0, 255);
// Print Red, Green, Blue values to serial monitor
Serial.print("Red:");
Serial.print(val1);
Serial.print("Green:");
Serial.print(val2);
Serial.print("Blue:");
Serial.println(val3);
colorRGB(val1, val2, val3);
// Display corresponding color on RGB LED
}
// Function to display a color
void colorRGB(int red, int green, int blue){
analogWrite(redPin, constrain(red, 0, 255));
// Adjust red intensity using PWM
analogWrite(greenPin, constrain(green, 0, 255));
// Adjust green intensity using PWM
analogWrite(bluePin, constrain(blue, 0, 255));
// Adjust blue intensity using PWM
}
After uploading the sketch, you can change different combinations of red, green and blue colors on the RGB LED.
License 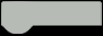
All Rights
Reserved
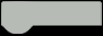
0
More from this category