In this tutorial, we will learn how to use an Arduino and a potentiometer to control the speed of a fan. A potentiometer is a variable resistor that can output an analog signal, the voltage of which changes as the potentiometer's knob is rotated.
COMPONENT LIST
HARDWARE

fig 1 Speed-Controlled Fan Circuit
CODE
Sample code:
// Project - Speed-Controlled Fan
// Define pin numbers
const int switchin = 0; // Input pin for the potentiometer
const int fanout = 3; // Output pin for the fan (using PWM)
int switchval = 0;
// Stores the analog value read from the potentiometer
int outputValue = 0;
// Stores the mapped value used to control fan speed
void setup() {
Serial.begin(9600); // Initialize serial communication
}
void loop() {
switchval = analogRead(switchin);
// Read the analog value from the potentiometer
Serial.println(switchval);
// Output the read value through the serial monitor
outputValue = map(switchval, 0, 1023, 0, 255);
// Map the analog value to a range of 0-255
/* This maps the 0-1023 range of the potentiometer to a 0-255 range for PWM control */
analogWrite(fanout, outputValue);
// Use PWM to control the fan speed
}
CODE REVIEW
Using a potentiometer to control fan speed is a typical input-calculate-output process control flow.
Input: read the analog value from the analog pin connected to the potentiometer:
analogRead(switchin)
Calculate: map the read analog value (ranging from 0-1023) to a new range (0-255), which is suitable for use with the analogWrite() function.
outputValue = map(switchval, 0, 1023, 0, 255)
Output: Control the speed of the fan connected to the fanout pin through PWM (Pulse Width Modulation) signals. The outputValue determines the duty cycle of the PWM signal, thereby regulating the speed of the fan.
analogWrite(fanout, outputValue)
The provided sample code is concise and easy to understand, for the rest, please refer to the comments for self-explanation.
HARDWARE
In previous projects, we learned how to use potentiometers to control the rotation of servos and the pitch of buzzers. In the hardware connections of projects such as [Interact with Servo] and [DJ Station] neither the servo nor the buzzer circuits incorporated additional electronic components. However, in this project, the small motor circuit incorporates an NPN transistor and a diode (as shown in the following diagram). Why can't the motor be directly connected to the circuit like the buzzer? We will then delve into the roles played by the NPN transistor and diode in the small motor circuit.

fig 2 130 Motor Driving Methods
The Role of NPN Transistors
In the Arduino UNO R3 main control board, each input/output pin has a maximum direct current (DC) rating of approximately 20mA, whereas a 130-sized small motor requires at least 100mA of current when not under load. Therefore, to control a DC motor with an Arduino, a method of amplifying the circuit's driving capability is necessary. This is where an NPN transistor comes into play (for more information on NPN transistors, refer to the project [Touch Switch].
Flyback (Freewheeling) Diode
The flyback diode, or freewheeling diode, protects circuits from voltage spikes and reverse voltage caused by inductive loads like motors. Inductive loads generate back electromotive force(back EMF) when current is suddenly interrupted which can damage other circuit components. Flyback diodes, connected in parallel with the inductive load, provide a safe path for current during such events preventing potential damage. The diode that plays this role is called a freewheeling diode. It's still a diode, but it just plays the role of freewheeling.
In the circuit of this project, when the 130 small motor is powered off, the coil will generate induced electromotive force (EMF) and reverse voltage, which may damage the components in the circuit (such as transistors). In order to prevent this risk, a diode needs to be connected in parallel at the two ends of the motor to absorb this reverse voltage and protect the circuit.
How to distinguish the positive and negative poles of electronic components? In this project, the diode schematic diagram is shown below. The section marked with a gray ring is the negative pole, and the other end is the positive pole.

fig 3 diode
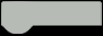