The focus of this project's learning is to understand and use the simple circuit-H bridge circuit to control the rotation and stop of the fan. Although the statements in the program are mostly basic and common Arduino statements, understanding how these statements combine with circuit principles is the key.
COMPONENT LIST
HARDWARE
Please connect the hardware according to Fig 1. This project uses a separate expansion breadboard. Pay attention to the connection direction of diodes, especially transistors.

fig 1 H-Bridge Motor Control
CODE
Sample code:
// Project - H-Bridge Motor Control
const int fanPin1 = 3;
const int fanPin2 = 5;
void setup() {
pinMode(fanPin1, OUTPUT);
pinMode(fanPin2, OUTPUT);
}
void loop() {
digitalWrite(fanPin1, HIGH);
digitalWrite(fanPin2, LOW);
delay(1000);
digitalWrite(fanPin1, LOW);
digitalWrite(fanPin2, HIGH);
delay(1000);
}
The fan will rotate in one direction for one second, then turn to the other direction and rotate for another second after uploading the program, . This process will repeat continuously, alternating between the two directions.
In this document, we first review the hardware components and their functions, followed by a detailed explanation of the code.
HARDWARE PREVIEW
H-Bridge Motor Control
The H-Bridge is a relatively simple circuit that typically comprises four independently controlled switching components (transistors). It is commonly used to drive high-current loads such as motors. As for why it is called an H-Bridge, it is because it resembles the letter H in appearance, as shown in Fig 2.
fig 2 H-Bridge Circuit
Here we have four transistors Q1, Q2, Q3, Q4, along with four flywheel diodes D1, D2, D3, D4, and a DC motor M. The four parallel flywheel diodes are used to prevent the spike voltage from the small motor from damaging the transistor components (see Project [Touch Switch] for details).
In this project's circuit, both NPN and PNP types of transistors are used. Both can be used as switches, but their base voltage requirements for conduction are different. NPN-type transistors can be considered as conducting at high voltage levels, while PNP-type transistors can be considered as conducting at low voltage levels. In this circuit, Q1 and Q3 are NPN-type and conduct at high voltage levels, while Q2 and Q4 are PNP-type and conduct at low voltage levels.

table 1 H-Bridge Motor Control Logic
(Note that the forward and reverse rotations in the above table depend on the connection direction of the positive and negative poles of the motor, and are specific to the hardware connection diagram of this project.)
The following CODE REVIEW will sort out the program in combination with the above hardware knowledge.
CODE REVIEW
In the loop() function:
digitalWrite(fanPin1, HIGH);
digitalWrite(fanPin2, LOW);
delay(1000);
digitalWrite(fanPin1, LOW);
digitalWrite(fanPin2, HIGH);
delay(1000);
By writing different signals to fanPin1 and fanPin2, the rotation of the motor can be controlled.
When fanPin1 is set to HIGH and fanPin2 is set to LOW, according to the working principle of the H-bridge circuit, the motor will rotate in one direction. delay(1000) keeps the motor rotating in this direction for 1 second.
Subsequently, fanPin1 is set to LOW and fanPin2 is set to HIGH, which will cause the motor to rotate in the opposite direction. Similarly, the motor maintains this direction of rotation for 1 second.
This process repeats continuously, causing the motor to alternate between rotating in two directions.
EXERCISE
The current implementation of this project results in the motor alternating its rotation between two directions. Notice that the motor also has a third state: the stopped state. Based on the H-Bridge Motor Control Logic table in the hardware section, let's attempt to modify the process so that the motor rotates for one second, stops for one second, and repeats this cycle continuously.
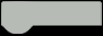