A sound-activated light is a highly practical smart home device that automatically turns on and off lighting based on detecting ambient sound. By programming the Arduino, we'll achieve functionality where the LED illuminates automatically when sound reaches a certain level and turns off once the sound diminishes.
COMPONENT LIST
HARDWARE
fig 1 Sound-Activated Light Circuit
CODE
Sample code:
// Project - Sound-Activated Light
// Define pins for the sound sensor and LED
const int soundSensorPin = A0;
// Connect the sound sensor to analog pin A0
const int ledPin = 13;
// Connect the LED to digital pin 13
const int soundThreshold = 500;
// The sound threshold value, adjustable based on the situation
void setup() {
pinMode(soundSensorPin, INPUT);
// Set the sound sensor pin as an input
pinMode(ledPin, OUTPUT); // Set the LED pin as an output
digitalWrite(ledPin, LOW); // Ensure the LED is off at startup
Serial.begin(9600);
// Initialize serial communication with a baud rate of 9600
}
void loop() {
// Read the value from the sound sensor
int sensorValue = analogRead(soundSensorPin);
// Print the value of the sound sensor through the serial monitor
Serial.print("Sound Sensor Value: ");
Serial.println(sensorValue);
// Check if the sound exceeds the set threshold
if (sensorValue > soundThreshold) {
digitalWrite(ledPin, HIGH);
// If the threshold is exceeded, turn on the LED
delay(1000); // Keep the LED on for 1 second
} else {
digitalWrite(ledPin, LOW);
// If the threshold is not exceeded, turn off the LED
}
// To avoid continuous checking, add a short delay
delay(50); // Delay for 50 milliseconds
}
After the code is uploaded successfully, when you make a loud noise, the LED will light up and stay on for one second. If you find it difficult to activate the LED (or it is too sensitive), you can adjust the sound threshold appropriately to achieve the best effect.
CODE REVIEW
Before the loop() function starts, it mainly performs some basic preparatory work, including initializing the pins used to control the LED, and setting serial communication parameters for data transmission or debugging.
In the loop() function of this project, it demonstrates a typical control flow of input, processing, and output.
Input, read the value from the sound sensor and store it in sensorValue :
int sensorValue = analogRead(soundSensorPin);
Processing, we will use an if statement to check if the sound value exceeds the preset threshold soundThreshold (process).
if (sensorValue > soundThreshold) {
digitalWrite(ledPin, HIGH);
delay(1000);
} else {
digitalWrite(ledPin, LOW);
}
If it exceeds the threshold, use digitalWrite(ledPin, HIGH) to light up the LED (output), and delay(1000) to keep the LED lit for one second. If it does not exceed the threshold, use digitalWrite(ledPin, LOW) to turn off the LED.
The function delay(50) is used to add a short delay between each sound detection, which helps reduce the load on the Arduino and improve the stability of the program.
HARDWARE REVIEW
Electret microphone
The basic principle of the electret microphone is a variable capacitor, and its capacitance value changes with sound vibration. In this way, the sound signal is converted into an electrical signal. In the circuit, it can be regarded as a resistor that varies with sound.
The electret microphone is polarized, so it has a positive pin and a ground pin:
fig 2 Electret Microphone Pin Diagram
The pin connected to the metal shell is the ground pin, and the other pin is the positive pin.
Audio signal created by microphone is a kind of alternating current, similar to the current in the power socket at home. But the alternating current is a sine wave with static frequency and fixed wavelength, while the audio wave is variable in both frequency and wavelength.
A higher frequency audio signal creates a higher pitched sound. And a lower frequency audio signal creates a lower pitched sound:
fig 3 Pitch
The “volume”, or “loudness” of a sound is directly related to the amplitude of the peaks. A higher amplitude audio signal creates a higher volume sound. And a lower amplitude audio signal creates a lower volume sound.

fig 4 Volume
DC bias
In order to ensure that the output signal of the microphone is in the required working range, we need to add a DC bias to it so that its output voltage signal is in the desired range.

fig 5 Working Range

fig 6 Circuit of Part with Microphone
Just like the fig 6, the essence of DC bias is to divide voltage between resistance R1 and microphone and then isolate DC part (the bias resistor generates DC voltage) by a capacitor C1.
PNP amplifying circuit
Next, let's look at the amplifier circuit, as shown in fig 7:
fig 7 Circuit of Part of amplifier
From the introduction of the NPN transistor in the project [Touch Switch], both NPN and PNP transistors can be used as switches and amplifiers. When a small change occurs at its base, the output signal will change significantly. This is the so-called amplification function. Because the voltage signal formed by the microphone through voltage division using R1 is relatively weak, a transistor amplification circuit is used to achieve better results.
In a PNP transistor amplification circuit, the base resistor R2 plays a biasing role, while the collector resistor R3 serves as a load element, achieving voltage amplification and conversion from current to voltage. These two resistors work together to ensure the normal operation of the amplification circuit and effective signal amplification.
So, what are the differences between PNP and NPN transistors when used in amplification circuits?
Due to the different material layers and directions of current flow in these two types of transistors, their working principles and characteristics in amplification circuits are different.

fig 8 NPN-type Transistor fig 9 PNP-type Transistor
(C: Collector; B: Base; E: Emitter)
As shown in fig 8 and fig 9: In the NPN circuit, C is connected to the positive pole, and E is connected to the negative pole; the PNP circuit is the opposite, with E connected to the positive pole and C connected to the negative pole. In general circuits, if NPN is used, you can get the PNP version by the method of "exchanging the upper and lower parts symmetrically".
Of course, to ensure normal operation, it is also necessary to ensure that these voltages and currents meet some quantitative conditions. The access position of the resistor needs to be adjusted to ensure the normal operation of the circuit and the effective amplification of the signal.
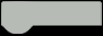