In this project, we will learn how to create an interesting interactive installation by combining an Arduino, a microphone, and a servo motor. The intensity of sound will be converted into the rotation angle of the servo motor through the Arduino, enabling the blades of the servo to rotate according to the volume of sound, thus visually demonstrating changes in sound.
COMPONENT LIST
HARDWARE
fig 1 Sound Level Meter Circuit
CODE
Sample Code:
// Project - Sound Level Meter
#include <Servo.h>
const int soundSensorPin = A0; // The sound sensor is connected to A0
Servo myservo; // Create a servo object
const float alpha = 0.1; /* Filter factor, between 0 and 1, smaller values result in stronger filtering*/
float filteredValue = 0; // Filtered value from the sound sensor
void setup() {
pinMode(soundSensorPin, INPUT);
Serial.begin(9600);
myservo.attach(13); // Attach the servo to digital pin 13
}
void loop() {
// Read the value from the sound sensor
int sensorValue = analogRead(soundSensorPin);
Serial.println(sensorValue); // Print the raw sensor value
// Apply the Exponential Moving Average filter
filteredValue = alpha * sensorValue + (1 - alpha) * filteredValue;
// Print the filtered sound sensor value through the Serial Monitor
Serial.print("Filtered sound value: ");
Serial.println(filteredValue);
/* Map the filtered sound value to the servo position range (typically 0 to 180 degrees)*/
int servoPos = map(filteredValue, 0, 1023, 0, 180);
// Control the servo to move to the specified position
myservo.write(servoPos);
// Delay for a short period to allow the servo to stabilize
delay(100);
}
After uploading the code, when you make a sound towards the microphone, the blade of the servo motor will rotate. Depending on the volume of the sound, showing different angles.
CODE REVIEW
Due to the instability and roughness of the signal transmitted by the microphone, when we directly use the signal on the servo, we will find that even if the sound does not change, the servo is still in a jittery state, resulting in an unstable implementation effect.
Therefore, when using an electret microphone module and a servo for volume display, it is essential to introduce a filtering mechanism, which can improve the smoothness and stability of the system and enhance user experience.
First, we need to define the filter factor (Alpha) value of the filter.
const float alpha = 0.1;
The Alpha value, which ranges from 0 to 1, determines the sensitivity of the filter to input data and the smoothness of the filtering effect.
filteredValue = alpha * sensorValue + (1 - alpha) * filteredValue;
The above calculation implements the Exponential Moving Average (EMA) filtering algorithm to smooth sound data.
Here, filterValues represent the filtered values that are the current, updated output values. These values change over time, taking into account both the latest input data (known as sensorValue) and the past filtered values. The filter factor, Alpha, determines how much weight is given to each of these. When Alpha is large, the filtered result reacts more quickly to the latest input data (sensorValue), closely following recent data changes. On the other hand, when Alpha is small, the filtered result becomes smoother, but it takes longer to respond to changes in the input data.
int servoPos = map(filteredValue, 0, 1023, 0, 180);
myservo.write(servoPos);
Finally, map the processed data to the angle servoPos within the working range of the servo, and use the myservo.write() function to present the sound loudness in the form of servo angle.
HARDWARE REVIEW
The hardware and circuitry used in this project are similar to those in the project titled [Sound-Activated Light]. If there are any uncertainties, please refer to the tutorial for the project [Sound-Activated Light].
EXERCISE
If the servo motor's rotation effect is not ideal during actual use, please try adjusting the value of the filtering factor to achieve smooth servo motor movement!
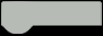