In this project, we will explore the fascinating world of joint programming between Processing and Arduino, realizing creative RGB lighting control through simple interface interactions. Imagine being able to remotely control the RGB LED light connected to the Arduino board simply by tapping buttons on the Processing interface, turning it on and off at will. This is not only a practice of programming skills but also an intriguing attempt to integrate digital creativity into our daily lives. Let's start and illuminate our lives with colors through code!
COMPONENT LIST
HARDWARE
Please connect the hardware according to the diagram below. For the pin diagram of the RGB LED, please refer to project [RGB LED] or [RGB Light Dimmer].
fig 1 Lighting Control Panel Circuit
CODE
Arduino Sample Code:
//Project - Lighting Control Panel
int redPin;
void setup() {
Serial.begin(9600);//enable serial communication
redPin = 9;
pinMode(redPin, OUTPUT);//define arduino pin
}
void loop() {
if (Serial.available() > 0) {//check serial value
char led = Serial.read();//read serial value
digitalWrite(redPin, LOW);
if (led == '1') {//check serial read
digitalWrite(redPin, HIGH);//redLED on
}else if (led == '2') {//check serial read
digitalWrite(redPin, LOW);//redLED off
}
}
}
Processing Sample Code:
//project - Lighting Control Panel
import processing.serial.*;//import serial communicate library
Serial myPort;//create serial object
int circleX, circleY; // Position of button
int circleSize = 100; // Diameter of button
color currentColor, baseColor;//color variable
color circleColor;//color variable
color circleHighlight;//color variable
boolean circleOver = false;
PFont f;//font type
void setup() {
size(600, 400);//screen size
circleColor = color(255,100,100);//set colors to variable
circleHighlight = color(200,50,50);//set colors to variable
baseColor = color(200);//set colors to variable
currentColor = baseColor;
circleX = width/4*2;//+circleSize/2+25;
circleY = height/2;
printArray(PFont.list());//print fonts list your computer
f = createFont("Ebrima Bold", 20);//Enter your font name
textFont(f);
String portName = "COM3";//Enter your COM port
myPort = new Serial(this, portName, 9600);//select first port
print(portName);
}
void draw() {
update(mouseX, mouseY);//main method
background(200);//background color
if (circleOver) {
fill(circleHighlight);
} else {
fill(circleColor);
}
stroke(255);
ellipse(circleX, circleY, circleSize, circleSize);//drow circle two
textAlign(CENTER);
fill(140,25,25);//red button
text("RED", width/4*2, height/2+10);//display text
}
void update(int x, int y) {
if (circleOver(circleX, circleY, circleSize) ) {
circleOver = true;
} else {
circleOver = false;
}
}
int clicknumber;
void mousePressed(){
if (circleOver) {
currentColor = circleColor;
clicknumber = clicknumber + 1;
if(clicknumber%2 == 1){
print("GreenON");
myPort.write('1');//send value zero
}
else{
myPort.write('2');//send value two
print("GreenOff");
}
}
}
boolean circleOver(int x, int y, int diameter) {
float disX = x - mouseX;
float disY = y - mouseY;
if (sqrt(sq(disX) + sq(disY)) < diameter/2 ) {
return true;
} else {
return false;
}
}
For this project, we will use Processing software in conjunction with the Arduino IDE to write programs that enable control of an Arduino via the Processing panel on a computer to execute corresponding operations. For instance, in this project, we will use buttons on the Processing panel to make an RGB color-changing light connected to the Arduino display corresponding colors.
Before writing the program, we need to download the Processing software. The download method is as follows:
Open a web browser and enter the website URL.
https://processing.org/download

Select the corresponding version to download.

On Windows, you'll have a .zip file.
Double-click it, and drag the folder inside to a location on your hard disk. But the important thing is for the processing folder to be pulled out of that .zip file. Then double-click processing.exe to start.

With any luck, the main Processing window will now be visible. Everyone's setup is different, so if the program didn't start, or you're otherwise stuck, visit the troubleshooting page for possible solutions.
Just click on "Get Started" to enter the Processing programming interface.

Your First Program
You're now running the Processing Development Environment (or PDE). There's not much to it; the large area is the Text Editor, and there's a row of buttons across the top; this is the toolbar. Below the editor is the Message Area, and below that is the Console. The Message Area is used for one line messages, and the Console is used for more technical details.

In the editor, type the following:
ellipse(50, 50, 80, 80);

This line of code means "draw an ellipse, with the center 50 pixels over from the left and 50 pixels down from the top, with a width and height of 80 pixels." Click the Run button the (triangle button in the Toolbar).

You'll see a circle on your screen if you've typed everything correctly.

If you didn't type it correctly, the Message Area will turn red and complain about an error.

If this happens, make sure that you've copied the example code exactly: the numbers should be contained within parentheses and have commas between each of them, and the line should end with a semicolon.
One of the most difficult things about getting started with programming is that you have to be very specific about the syntax. The Processing software isn't always smart enough to know what you mean, and can be quite fussy about the placement of punctuation. You'll get used to it with a little practice.
CODE REVIEW
The interaction between the Arduino IDE and Processing is primarily achieved through Serial Communication, enabling Arduino to control hardware devices (such as RGB LEDs) and send data to the computer, while Processing can receive this data for visualization or send control commands to Arduino.
Depending on the received instructions, Arduino performs corresponding operations.
Although the Processing code and Arduino code in the code example section are two separate programs, they are not completely isolated but rather work together for operation and use. Therefore, during the explanation of the code below, although the two programs will not be interspersed for the sake of continuity and completeness of the explanation, please refer to both sets of codes simultaneously for understanding. This will help you better grasp the logical principles of the code.
processing
size(600, 400);
circleColor = color(255, 100, 100);
circleHighlight = color(200, 50, 50);
baseColor = color(200);
currentColor = baseColor;
circleX = width / 4 * 2;
circleY = height / 2;
printArray(PFont.list());
f = createFont("Ebrima Bold", 20);
textFont(f);
String portName = "COM3";
myPort = new Serial(this, portName, 9600);
print(portName);
In the setup() function, the screen size, button position, colors, and fonts are set up.
Importantly, a serial communication connection with Arduino is established by inputting the device port number connected to Arduino using String portName = "COM3";, and the serial communication is initialized.
The draw() function is used to draw all elements on the screen.
update(mouseX, mouseY);
The update() function is used to determine whether the mouse is hovering over a button.
if (circleOver) {
fill(circleHighlight);
} else {
fill(circleColor);
}
Using an if-else statement to update the button color: when the mouse hovers over the button area, it displays a highlighted color; otherwise, it displays the button's original color.
circle(circleX, circleY, circleSize);
stroke(255);
fill(140, 25, 25);
textAlign(CENTER);
text("RED", width / 4 * 2, height / 2 + 10);
The circle(x, y, d) function is used to draw a circle (which serves as a button in conjunction with corresponding functions), where x and y represent the position of the circle's center, and d corresponds to the circle's diameter. Additionally, the border of the circle, as well as the color and position of the text content, are drawn.
The update() function updates the hover state of each button based on the current mouse position (x, y).
void update(int x, int y) {
if (circleOver(circleX, circleY, circleSize)) {
circleOver = true;
} else {
circleOver = false;
}
}
Although it is named update, it is actually a function called within the draw() function to update the hover state without directly drawing any content. Within this function, circleOver(circleX, circleY, circleSize) is a helper method of the update() function used to determine whether the mouse is hovering over the circular area of the button.
boolean circleOver(int x, int y, int diameter) {
float disX = x - mouseX;
float disY = y - mouseY;
if (sqrt(sq(disX) + sq(disY)) < diameter / 2) {
return true;
} else {
return false;
}
}
These helper methods are used to determine whether the mouse is hovering over a specific circular button.
disX and disY are the differences between the current mouse position and the horizontal and vertical coordinates of the button's center (these differences can be positive or negative, but only the square values of these differences are used subsequently, so the sign does not matter). Using the Pythagorean theorem a²+b²=c², the distance between the mouse position and the button center is calculated using sqrt(sq(disX) + sq(disY)). If the distance is less than the button's radius, it returns true, indicating that the mouse is hovering over the button. Otherwise, it returns false, indicating that the mouse is hovering outside the button.
Finally, the mousePressed() function is a built-in event handling function in Processing that is called when the user clicks the mouse.
void mousePressed() {
if (circleOver) {
clicknumber = clicknumber + 1;
if (clicknumber % 2 == 1) {
print("RedON");
myPort.write('1');
background(200);
} else {
myPort.write('2');
print("RedOff");
background(50);
}
}
}
When the user clicks the mouse, within the mousePressed() function, a check is performed to determine if the click occurred inside a circular button. If so, the button press count clicknumber is incremented by 1. Next, a judgment is made based on the total number of presses: if the number of presses is odd, the digit "1" is sent to Arduino; otherwise, if even, the digit "2" is sent. Corresponding switch information is also output to the console.
The Processing program does not directly control the high and low levels of the RGB LED but instead sends digital signals to Arduino. Arduino, based on the received digital signals, outputs corresponding high and low levels to the RGB LED to control its color and brightness. In this way, Processing and Arduino work together to indirectly control the RGB LED.
Arduino
In the loop() function, if (Serial.available() > 0) checks whether there is new data available to read from the serial port. If there is, the code within the curly braces is executed.
if (Serial.available() > 0) {
}
Within the if execution block, the number sent from Processing is first assigned to a string variable named led. The next two if statements then check the value of the read data led. If it is '1', the LED is turned on using digitalWrite(redPin, HIGH); if it is '2', the LED is turned off using digitalWrite(redPin, LOW).
char led = Serial.read();
if (led == '1') {
digitalWrite(redPin, HIGH);
} else if (led == '2') {
digitalWrite(redPin, LOW);
}
EXERCISE
Utilize multiple buttons to adjust the brightness or change the color of the RGB LED.
Unleash your imagination and create a powerful dimming panel to achieve even more colorful lighting effects!
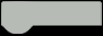